Computer Science
A square matrix is the matrix in which number of rows equals the number of columns. Thus, a matrix of order n*n is called a Square Matrix.
Write a program in Java to create a double dimensional array of size nxn matrix form and fill the numbers in a circular fashion (anticlock-wise) with natural numbers from 1 to n2, taking n as an input. The filling of the elements should start from outer to the central cell.
For example, if n=4, then n2=16, then the array is filled as:
1 ↓ | ↓ 12 | ← 11 | ← 10 |
2 ↓ | ↓ 13 | 16 ↑ | 9 ↑ |
3 ↓ | 14 → | 15 ↑ | 8 ↑ |
4 → | 5 → | 6 → | 7 ↑ |
Java
Java Arrays
11 Likes
Answer
import java.util.Scanner;
public class KboatMatrixCircularFill
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the size of the matrix: ");
int n = in.nextInt();
int x = 1;
int arr[][] = new int[n][n];
int a = 0;
int b = n - 1;
while (a < n) {
for (int i = a; i <= b; i++) {
arr[i][a] = x++;
}
a++;
for (int i = a; i <= b; i++) {
arr[b][i] = x++;
}
for (int i = b - 1; i >= a - 1; i--) {
arr[i][b] = x++;
}
b--;
for (int i = b; i >= a; i--) {
arr[a-1][i] = x++;
}
}
System.out.println("Circular Matrix Anti-Clockwise:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
}
Output
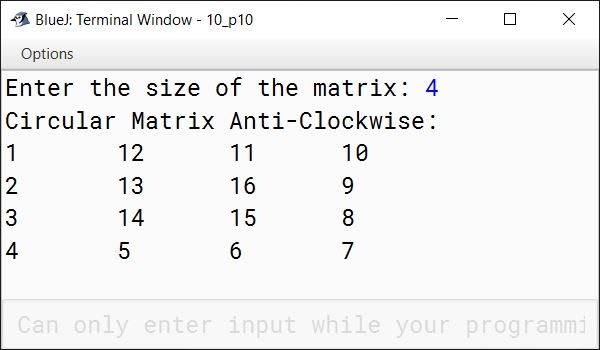
Answered By
5 Likes
Related Questions
Write a Program in Java to input elements in a 2D square matrix and check whether it is a Lower Triangular Matrix or not.
Lower Triangular Matrix: A Lower Triangular matrix is a square matrix in which all the entries above the main diagonal [] are zero. The entries below or on the main diagonal must be non zero values.
Enter the size of the matrix: 4
The Matrix is:
5 0 0 0
3 1 0 0
4 9 4 0
6 8 7 2The Matrix is Lower Triangular
Write a Program in Java to input elements in a 2-D square matrix and check whether it is a Scalar Matrix or not.
Scalar Matrix: A scalar matrix is a diagonal matrix where the left diagonal elements are same.
The Matrix is:
5 0 0 0
0 5 0 0
0 0 5 0
0 0 0 5The Matrix is Scalar
A square matrix is the matrix in which number of rows equals the number of columns. Thus, a matrix of order n*n is called a Square Matrix.
Write a program in Java to create a double dimensional array of size nxn matrix form and fill the cells of matrix in a circular fashion (clock wise) with natural numbers from 1 to n2, taking n as an input. Input n should be an odd number and filling of the elements should start from the central cell.
For example, if n = 5, then n2 = 25, then the array is filled as:
21 22 23 24 25 20 7 8 9 10 19 6 1 2 11 18 5 4 3 12 17 16 15 14 13 Write a program in Java create a double dimensional array of size nxn matrix form and fill the numbers in a circular fashion (anticlock-wise) with natural numbers from 1 to n2, as illustrated below:
For example, if n = 5, then n2 = 25, then the array is filled as:
21 20 19 18 17 22 7 6 5 16 23 8 1 4 15 24 9 2 3 14 25 10 11 12 13