Computer Science
The result of a quiz competition is to be prepared as follows:
The quiz has five questions with four multiple choices (A, B, C, D), with each question carrying 1 mark for the correct answer. Design a program to accept the number of participants N such that N must be greater than 3 and less than 11. Create a double-dimensional array of size (Nx5) to store the answers of each participant row-wise. Calculate the marks for each participant by matching the correct answer stored in a single-dimensional array of size 5. Display the scores for each participant and also the participant(s) having the highest score.
Example: If the value of N = 4, then the array would be:
Q1 | Q2 | Q3 | Q4 | Q5 | |
---|---|---|---|---|---|
Participant 1 | A | B | B | C | A |
Participant 2 | D | A | D | C | B |
Participant 3 | A | A | B | A | C |
Participant 4 | D | C | C | A | B |
Key to the question: | D | C | C | B | A |
Note: Array entries are line fed (i.e. one entry per line)
Test your program for the following data and some random data.
Example 1
INPUT:
N = 5
Participant 1 D A B C C
Participant 2 A A D C B
Participant 3 B A C D B
Participant 4 D A D C B
Participant 5 B C A D D
Key: B C D A A
OUTPUT:
Scores:
Participant 1 = 0
Participant 2 = 1
Participant 3 = 1
Participant 4 = 1
Participant 5 = 2
Highest Score:
Participant 5
Example 2
INPUT:
N = 4
Participant 1 A C C B D
Participant 2 B C A A C
Participant 3 B C B A A
Participant 4 C C D D B
Key: A C D B B
OUTPUT:
Scores:
Participant 1 = 3
Participant 2 = 1
Participant 3 = 1
Participant 4 = 3
Highest Score:
Participant 1
Participant 4
Example 3
INPUT:
N = 12
OUTPUT:
INPUT SIZE OUT OF RANGE.
Java
Java Arrays
ICSE Prac 2017
10 Likes
Answer
import java.util.Scanner;
public class QuizCompetition
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the Number of Participants (N): ");
int n = in.nextInt();
if (n <= 3 || n >= 11) {
System.out.println("INPUT SIZE OUT OF RANGE.");
return;
}
char answers[][] = new char[n][5];
char key[] = new char[5];
System.out.println("Enter answers of participants");
for (int i = 0; i < n; i++) {
System.out.println("Participant " + (i+1));
for (int j = 0; j < 5; j++) {
answers[i][j] = in.next().charAt(0);
}
}
System.out.println("Enter Answer Key:");
for (int i = 0; i < 5; i++) {
key[i] = in.next().charAt(0);
}
int hScore = 0;
int score[] = new int[n];
System.out.println("Scores:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < 5; j++) {
if (answers[i][j] == key[j]) {
score[i]++;
}
}
if (score[i] > hScore) {
hScore = score[i];
}
System.out.println("Participant " + (i+1) + " = " + score[i]);
}
System.out.println("Highest Score:");
for (int i = 0; i < n; i++) {
if (score[i] == hScore) {
System.out.println("Participant " + (i+1));
}
}
}
}
Output
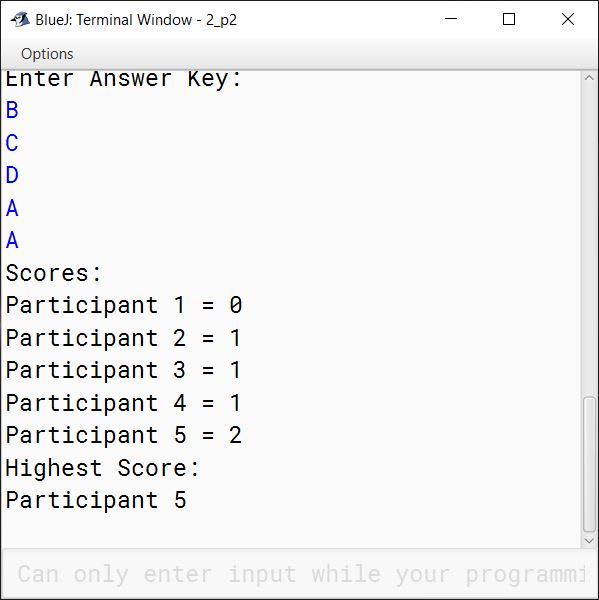
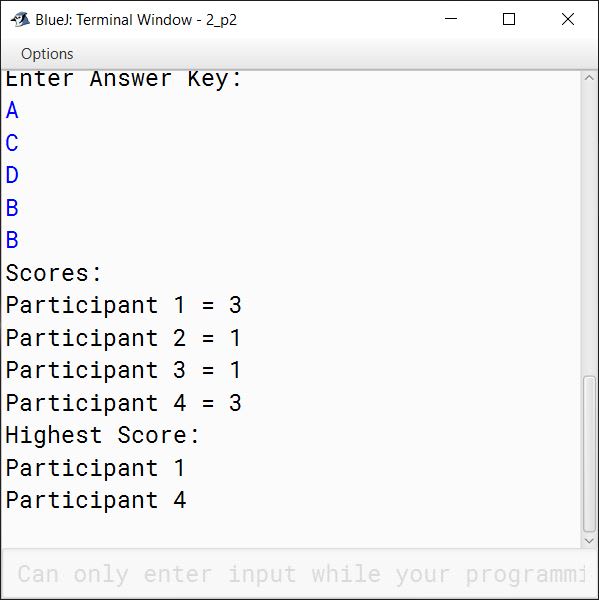
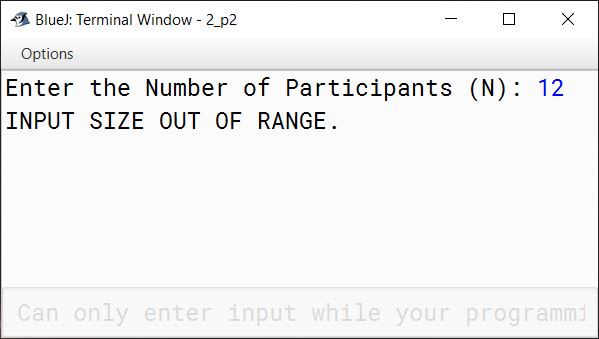
Answered By
3 Likes