Computer Applications
Write a program in Java to accept a word/a String and display the new string after removing all the vowels present in it.
Sample Input: COMPUTER APPLICATIONS
Sample Output: CMPTR PPLCTNS
Java
Java String Handling
207 Likes
Answer
import java.util.Scanner;
public class KboatVowelRemoval
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a word or sentence:");
String str = in.nextLine();
int len = str.length();
String newStr = "";
for (int i = 0; i < len; i++) {
char ch = Character.toUpperCase(str.charAt(i));
if (ch == 'A' ||
ch == 'E' ||
ch == 'I' ||
ch == 'O' ||
ch == 'U') {
continue;
}
newStr = newStr + ch;
}
System.out.println("String with vowels removed");
System.out.println(newStr);
}
}
Variable Description Table
Program Explanation
Output
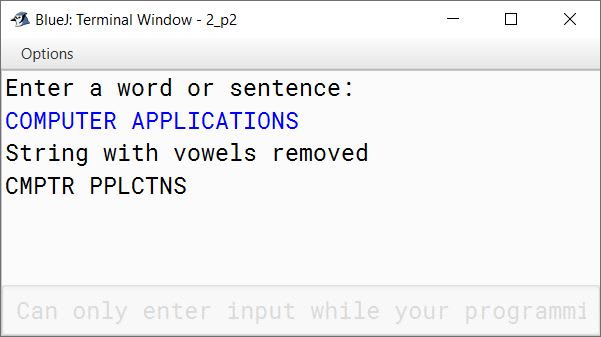
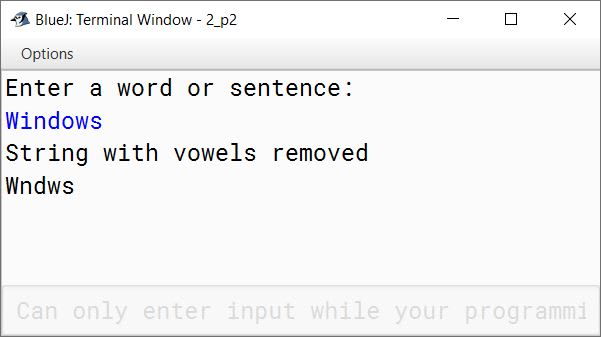
Answered By
80 Likes
Related Questions
Write a program in Java to accept a name(Containing three words) and Display only the initials (i.e., first letter of each word).
Sample Input: LAL KRISHNA ADVANI
Sample Output: L K AWrite a program in Java to enter a String/Sentence and display the longest word and the length of the longest word present in the String.
Sample Input: “TATA FOOTBALL ACADEMY WILL PLAY AGAINST MOHAN BAGAN”
Sample Output: The longest word: FOOTBALL: The length of the word: 8Write a program to input a sentence. Find and display the following:
(i) Number of words present in the sentence
(ii) Number of letters present in the sentence
Assume that the sentence has neither include any digit nor a special character.Write a program in Java to accept a name containing three words and display the surname first, followed by the first and middle names.
Sample Input: MOHANDAS KARAMCHAND GANDHI
Sample Output: GANDHI MOHANDAS KARAMCHAND