Computer Applications
Write a program to input a sentence. Find and display the following:
(i) Number of words present in the sentence
(ii) Number of letters present in the sentence
Assume that the sentence has neither include any digit nor a special character.
Java
Java String Handling
244 Likes
Answer
import java.util.Scanner;
public class KboatWordsNLetters
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
int wCount = 0, lCount = 0;
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == ' ')
wCount++;
else
lCount++;
}
/*
* Number of words in a sentence are one more than
* the number of spaces so incrementing wCount by 1
*/
wCount++;
System.out.println("No. of words = " + wCount);
System.out.println("No. of letters = " + lCount);
}
}
Variable Description Table
Program Explanation
Output
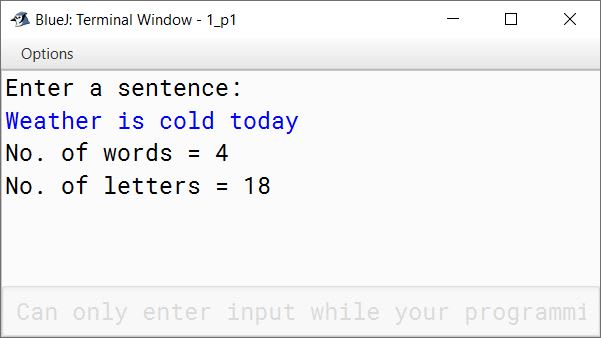
Answered By
110 Likes
Related Questions
Describe the purpose and write the syntax of startWith() function.
Describe the purpose and write the syntax of equalsIgnoreCase() function.
Write a program in Java to accept a word/a String and display the new string after removing all the vowels present in it.
Sample Input: COMPUTER APPLICATIONS
Sample Output: CMPTR PPLCTNSWrite a program in Java to accept a name(Containing three words) and Display only the initials (i.e., first letter of each word).
Sample Input: LAL KRISHNA ADVANI
Sample Output: L K A