Computer Applications
Write a program in Java to accept a name containing three words and display the surname first, followed by the first and middle names.
Sample Input: MOHANDAS KARAMCHAND GANDHI
Sample Output: GANDHI MOHANDAS KARAMCHAND
Java
Java String Handling
155 Likes
Answer
import java.util.Scanner;
public class KboatSurnameFirst
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a name of 3 words:");
String name = in.nextLine();
/*
* Get the last index
* of space in the string
*/
int lastSpaceIdx = name.lastIndexOf(' ');
String surname = name.substring(lastSpaceIdx + 1);
String initialName = name.substring(0, lastSpaceIdx);
System.out.println(surname + " " + initialName);
}
}
Variable Description Table
Program Explanation
Output
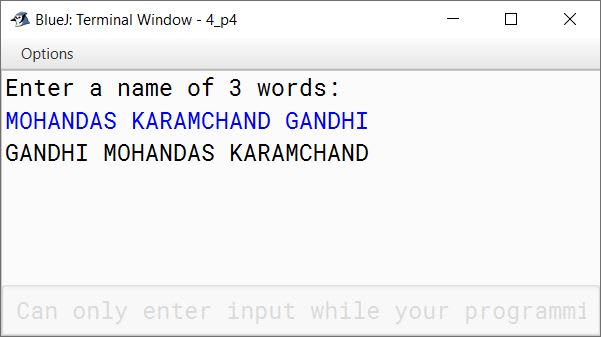
Answered By
70 Likes
Related Questions
Write a program in Java to accept a word and display the ASCII code of each character of the word.
Sample Input: BLUEJ
Sample Output:
ASCII of B = 66
ASCII of L = 76
ASCII of U = 85
ASCII of E = 69
ASCII of J = 74Write a program in Java to accept a name(Containing three words) and Display only the initials (i.e., first letter of each word).
Sample Input: LAL KRISHNA ADVANI
Sample Output: L K AWrite a program in Java to enter a String/Sentence and display the longest word and the length of the longest word present in the String.
Sample Input: “TATA FOOTBALL ACADEMY WILL PLAY AGAINST MOHAN BAGAN”
Sample Output: The longest word: FOOTBALL: The length of the word: 8Write a program in Java to accept a word/a String and display the new string after removing all the vowels present in it.
Sample Input: COMPUTER APPLICATIONS
Sample Output: CMPTR PPLCTNS