Computer Applications
Write a program by using class 'Employee' to accept Basic Pay of an employee. Calculate the allowances/deductions as given below.
Allowance / Deduction | Rate |
---|---|
Dearness Allowance (DA) | 30% of Basic Pay |
House Rent Allowance (HRA) | 15% of Basic Pay |
Provident Fund (PF) | 12.5% of Basic Pay |
Finally, find and print the Gross and Net pay.
Gross Pay = Basic Pay + Dearness Allowance + House Rent Allowance
Net Pay = Gross Pay - Provident Fund
Java
Input in Java
ICSE 2005
269 Likes
Answer
import java.util.Scanner;
public class Employee
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Basic Pay: ");
double bp = in.nextDouble();
double da = 0.3 * bp;
double hra = 0.15 * bp;
double pf = 0.125 * bp;
double gp = bp + da + hra;
double np = gp - pf;
System.out.println("Gross Pay = " + gp);
System.out.println("Net Pay = " + np);
}
}
Variable Description Table
Program Explanation
Output
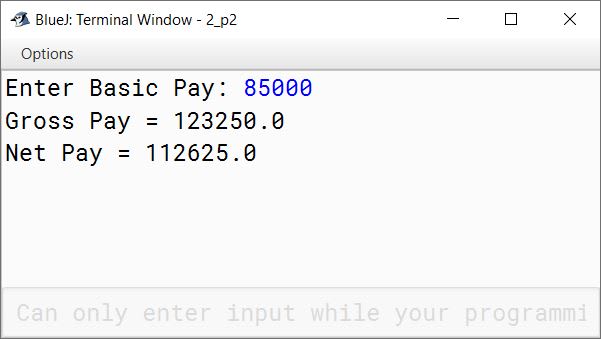
Answered By
123 Likes
Related Questions
Mr. Agarwal invests certain sum at 5% per annum compound interest for three years. Write a program in Java to calculate:
(a) the interest for the first year
(b) the interest for the second year
(c) the amount after three years.Take sum as an input from the user.
Sample Input: Principal = ₹5000, Rate =10%, Time = 3 yrs
Sample Output: Interest for the first year: ₹500
Interest for the second year: ₹550
Interest for the third year: ₹605A shopkeeper offers 10% discount on the printed price of a Digital Camera. However, a customer has to pay 6% GST on the remaining amount. Write a program in Java to calculate the amount to be paid by the customer taking printed price as an input.
A shopkeeper offers 30% discount on purchasing articles whereas the other shopkeeper offers two successive discounts 20% and 10% for purchasing the same articles. Write a program in Java to compute and display the discounts.
Take the price of an article as the input.The time period of a Simple Pendulum is given by the formula:
T = 2π√(l/g)
Write a program to calculate the time period of a Simple Pendulum by taking length and acceleration due to gravity (g) as inputs.