Computer Applications
Mr. Agarwal invests certain sum at 5% per annum compound interest for three years. Write a program in Java to calculate:
(a) the interest for the first year
(b) the interest for the second year
(c) the amount after three years.
Take sum as an input from the user.
Sample Input: Principal = ₹5000, Rate =10%, Time = 3 yrs
Sample Output: Interest for the first year: ₹500
Interest for the second year: ₹550
Interest for the third year: ₹605
Java
Input in Java
167 Likes
Answer
import java.util.Scanner;
public class KboatCompoundInterest
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter sum of money: ");
double p = in.nextDouble();
double interest = p * 5 * 1 / 100.0;
System.out.println("Interest for the first year = " + interest);
p += interest;
interest = p * 5 * 1 / 100.0;
System.out.println("Interest for the second year = " + interest);
p += interest;
interest = p * 5 * 1 / 100.0;
System.out.println("Interest for the third year = " + interest);
}
}
Variable Description Table
Program Explanation
Output
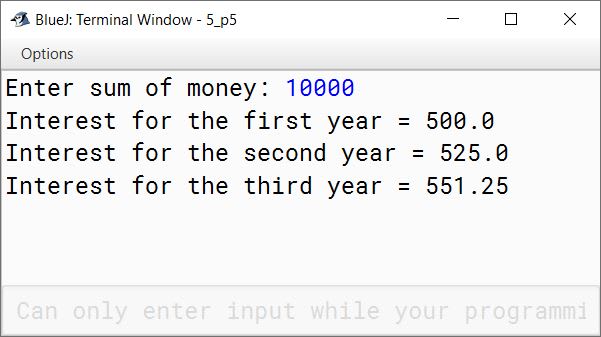
Answered By
74 Likes
Related Questions
A shopkeeper offers 10% discount on the printed price of a Digital Camera. However, a customer has to pay 6% GST on the remaining amount. Write a program in Java to calculate the amount to be paid by the customer taking printed price as an input.
Write a program to input time in seconds. Display the time after converting them into hours, minutes and seconds.
Sample Input: Time in seconds: 5420
Sample Output: 1 Hour 30 Minutes 20 Seconds
A businessman wishes to accumulate 3000 shares of a company. However, he already has some shares of that company valuing ₹10 (nominal value) which yield 10% dividend per annum and receive ₹2000 as dividend at the end of the year. Write a program in Java to calculate the number of shares he has and how many more shares to be purchased to make his target.
Hint: No. of share = (Annual dividend * 100) / (Nominal value * div%)A shopkeeper offers 30% discount on purchasing articles whereas the other shopkeeper offers two successive discounts 20% and 10% for purchasing the same articles. Write a program in Java to compute and display the discounts.
Take the price of an article as the input.