Computer Applications
A shopkeeper offers 30% discount on purchasing articles whereas the other shopkeeper offers two successive discounts 20% and 10% for purchasing the same articles. Write a program in Java to compute and display the discounts.
Take the price of an article as the input.
Java
Input in Java
233 Likes
Answer
import java.util.Scanner;
public class KboatDiscounts
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter price of article: ");
double price = in.nextDouble();
double d1 = price * 30 / 100.0;
double amt1 = price - d1;
System.out.println("30% discount = " + d1);
System.out.println("Amount after 30% discount = " + amt1);
double d2 = price * 20 / 100.0;
double amt2 = price - d2;
double d3 = amt2 * 10 / 100.0;
amt2 -= d3;
System.out.println("20% discount = " + d2);
System.out.println("10% discount = " + d3);
System.out.println("Amount after successive discounts = " + amt2);
}
}
Variable Description Table
Program Explanation
Output
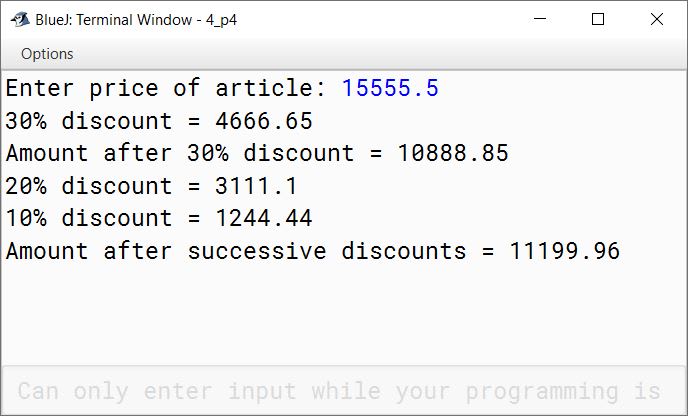
Answered By
94 Likes
Related Questions
Write a program by using class 'Employee' to accept Basic Pay of an employee. Calculate the allowances/deductions as given below.
Allowance / Deduction Rate Dearness Allowance (DA) 30% of Basic Pay House Rent Allowance (HRA) 15% of Basic Pay Provident Fund (PF) 12.5% of Basic Pay Finally, find and print the Gross and Net pay.
Gross Pay = Basic Pay + Dearness Allowance + House Rent Allowance
Net Pay = Gross Pay - Provident FundA businessman wishes to accumulate 3000 shares of a company. However, he already has some shares of that company valuing ₹10 (nominal value) which yield 10% dividend per annum and receive ₹2000 as dividend at the end of the year. Write a program in Java to calculate the number of shares he has and how many more shares to be purchased to make his target.
Hint: No. of share = (Annual dividend * 100) / (Nominal value * div%)A shopkeeper offers 10% discount on the printed price of a Digital Camera. However, a customer has to pay 6% GST on the remaining amount. Write a program in Java to calculate the amount to be paid by the customer taking printed price as an input.
Mr. Agarwal invests certain sum at 5% per annum compound interest for three years. Write a program in Java to calculate:
(a) the interest for the first year
(b) the interest for the second year
(c) the amount after three years.Take sum as an input from the user.
Sample Input: Principal = ₹5000, Rate =10%, Time = 3 yrs
Sample Output: Interest for the first year: ₹500
Interest for the second year: ₹550
Interest for the third year: ₹605