Computer Applications
Write a program by using a class with the following specifications:
Class name: Vowel
Data Members | Purpose |
---|---|
String s | To store the string |
int c | To count vowels |
Member Methods | Purpose |
---|---|
void getstr() | to accept a string |
void getvowel() | to count the number of vowels |
void display() | to print the number of vowels |
Java
Java Classes
20 Likes
Answer
import java.util.Scanner;
public class Vowel
{
private String s;
private int c;
public void getstr() {
Scanner in = new Scanner(System.in);
System.out.print("Enter the string: ");
s = in.nextLine();
}
public void getvowel() {
String temp = s.toUpperCase();
c = 0;
for (int i = 0; i < temp.length(); i++) {
char ch = temp.charAt(i);
if (ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O'
|| ch == 'U')
c++;
}
}
public void display() {
System.out.println("No. of Vowels = " + c);
}
public static void main(String args[]) {
Vowel obj = new Vowel();
obj.getstr();
obj.getvowel();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
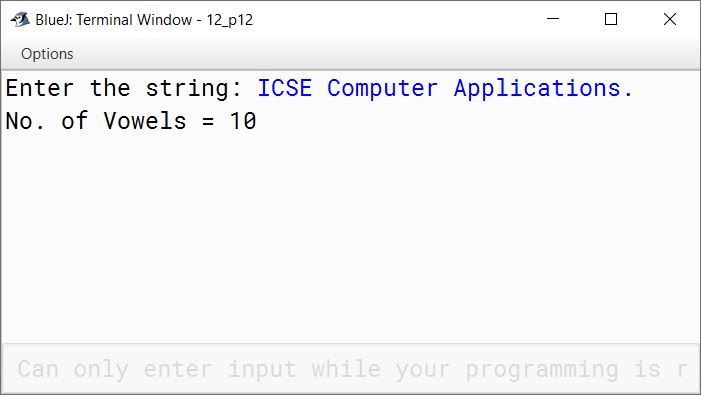
Answered By
9 Likes
Related Questions
Define a class called 'Mobike' with the following specifications:
Data Members Purpose int bno To store the bike number int phno To store the phone number of the customer String name To store the name of the customer int days To store the number of days the bike is taken on rent int charge To calculate and store the rental charge Member Methods Purpose void input() To input and store the details of the customer void compute() To compute the rental charge void display() To display the details in the given format The rent for a mobike is charged on the following basis:
Days Charge For first five days ₹500 per day For next five days ₹400 per day Rest of the days ₹200 per day Output:
Bike No. Phone No. Name No. of days Charge xxxxxxx xxxxxxxx xxxx xxx xxxxxx
Write a program using a class with the following specifications:
Class name: Caseconvert
Data Members Purpose String str To store the string Member Methods Purpose void getstr() to accept a string void convert() to obtain a string after converting each upper case letter into lower case and vice versa void display() to print the converted string A bookseller maintains record of books belonging to the various publishers. He uses a class with the specifications given below:
Class name — Stock
Data Members:
- String title — Contains title of the book
- String author — Contains author name
- String pub — Contains publisher's name
- int noc — Number of copies
Member Methods:
- void getdata() — To accept title, author, publisher's name and the number of copies.
- void purchase(int t, String a, String p, int n) — To check the existence of the book in the stock by comparing total, author's and publisher's name. Also check whether noc >n or not. If yes, maintain the balance as noc-n, otherwise display book is not available or stock is under flowing.
Write a program to perform the task given above.
Write a program by using class with the following specifications:
Class name — Characters
Data Members:
- String str — To store the string
Member Methods:
- void input (String st) — to assign st to str
- void check_print() — to check and print the following:
(i) number of letters
(ii) number of digits
(iii) number of uppercase characters
(iv) number of lowercase characters
(v) number of special characters