Computer Applications
Write a menu driven program to perform the following tasks by using Switch case statement:
(a) To print the series:
0, 3, 8, 15, 24, ………… to n terms. (value of 'n' is to be an input by the user)
(b) To find the sum of the series:
S = (1/2) + (3/4) + (5/6) + (7/8) + ……….. + (19/20)
Java
Java Iterative Stmts
ICSE 2011
181 Likes
Answer
import java.util.Scanner;
public class KboatSeriesMenu
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 to print series");
System.out.println("0, 3, 8, 15, 24,....to n terms");
System.out.println();
System.out.println("Type 2 to find sum of series");
System.out.println("(1/2) + (3/4) + (5/6) + (7/8) +....+ (19/20)");
System.out.println();
System.out.print("Enter your choice: ");
int choice = in.nextInt();
switch (choice) {
case 1:
System.out.print("Enter n: ");
int n = in.nextInt();
for (int i = 1; i <= n; i++)
System.out.print(((i * i) - 1) + " ");
System.out.println();
break;
case 2:
double sum = 0;
for (int i = 1; i <= 19; i = i + 2)
sum += i / (double)(i + 1);
System.out.println("Sum = " + sum);
break;
default:
System.out.println("Incorrect Choice");
break;
}
}
}
Variable Description Table
Program Explanation
Output
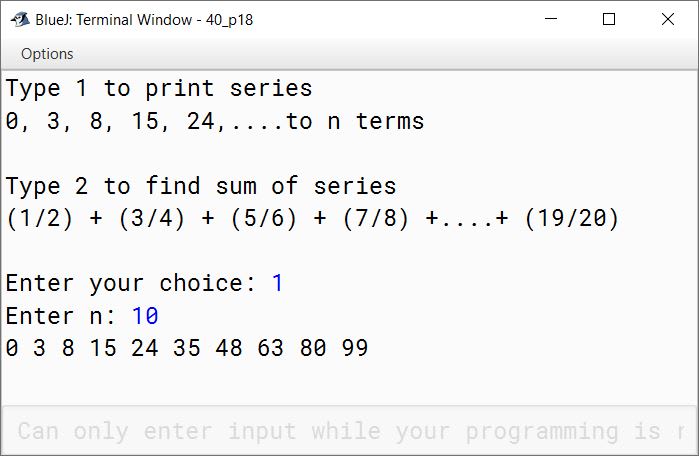
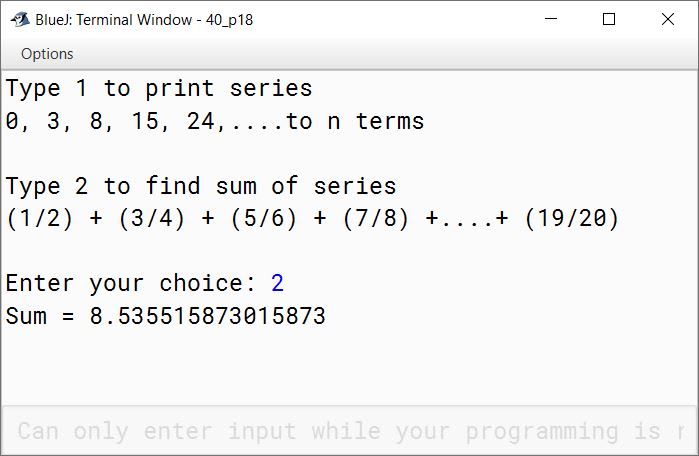
Answered By
52 Likes
Related Questions
A special two-digit number is such that when the sum of its digits is added to the product of its digits, the result is equal to the original two-digit number.
Example: Consider the number 59.
Sum of digits = 5 + 9 = 14
Product of digits = 5 * 9 = 45
Sum of the sum of digits and product of digits = 14 + 45 = 59Write a program to accept a two-digit number. Add the sum of its digits to the product of its digits. If the value is equal to the number input, then display the message "Special two—digit number" otherwise, display the message "Not a special two-digit number".
Write a menu driven class to accept a number from the user and check whether it is a Palindrome or a Perfect number.
(a) Palindrome number: (A number is a Palindrome which when read in reverse order is same as in the right order)
Example: 11, 101, 151 etc.
(b) Perfect number: (A number is called Perfect if it is equal to the sum of its factors other than the number itself.)
Example: 6 = 1 + 2 + 3
Using a switch statement, write a menu driven program to:
(a) Generate and display the first 10 terms of the Fibonacci series
0, 1, 1, 2, 3, 5
The first two Fibonacci numbers are 0 and 1, and each subsequent number is the sum of the previous two.
(b) Find the sum of the digits of an integer that is input.
Sample Input: 15390
Sample Output: Sum of the digits = 18For an incorrect choice, an appropriate error message should be displayed.
Write a menu driven program to accept a number from the user and check whether it is a Prime number or an Automorphic number.
(a) Prime number: (A number is said to be prime, if it is only divisible by 1 and itself)
Example: 3,5,7,11
(b) Automorphic number: (Automorphic number is the number which is contained in the last digit(s) of its square.)
Example: 25 is an Automorphic number as its square is 625 and 25 is present as the last two digits.