Computer Applications
A special two-digit number is such that when the sum of its digits is added to the product of its digits, the result is equal to the original two-digit number.
Example: Consider the number 59.
Sum of digits = 5 + 9 = 14
Product of digits = 5 * 9 = 45
Sum of the sum of digits and product of digits = 14 + 45 = 59
Write a program to accept a two-digit number. Add the sum of its digits to the product of its digits. If the value is equal to the number input, then display the message "Special two—digit number" otherwise, display the message "Not a special two-digit number".
Java
Java Iterative Stmts
ICSE 2014
301 Likes
Answer
import java.util.Scanner;
public class KboatSpecialNumber
{
public void checkNumber() {
Scanner in = new Scanner(System.in);
System.out.print("Enter a 2 digit number: ");
int orgNum = in.nextInt();
int num = orgNum;
int count = 0, digitSum = 0, digitProduct = 1;
while (num != 0) {
int digit = num % 10;
num /= 10;
digitSum += digit;
digitProduct *= digit;
count++;
}
if (count != 2)
System.out.println("Invalid input, please enter a 2-digit number");
else if ((digitSum + digitProduct) == orgNum)
System.out.println("Special 2-digit number");
else
System.out.println("Not a special 2-digit number");
}
}
Variable Description Table
Program Explanation
Output
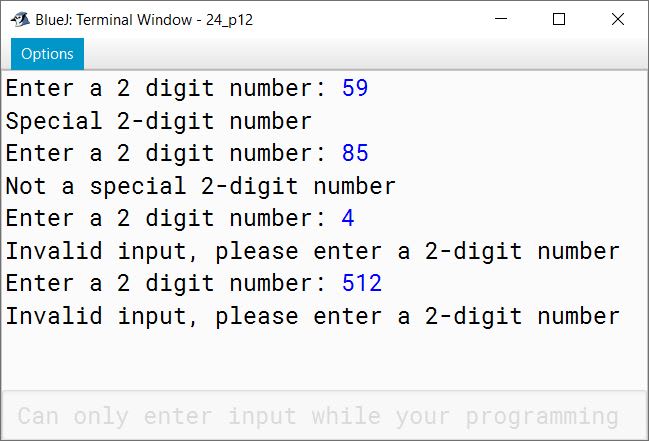
Answered By
117 Likes
Related Questions
Write a program to input a number and display it into its Binary equivalent.
Sample Input: (21)10
Sample Output: (10101)2An abundant number is a number for which the sum of its proper divisors (excluding the number itself) is greater than the original number. Write a program to input number and check whether it is an abundant number or not.
Sample input: 12
Sample output: It is an abundant number.
Explanation: Its proper divisors are 1, 2, 3, 4 and 6
Sum = 1 + 2 + 3 + 4 + 6 = 16
Hence, 12 is an abundant number.Write a program to accept a number and check whether it is a 'Spy Number' or not. (A number is spy if the sum of its digits equals the product of its digits.)
Example: Sample Input: 1124
Sum of the digits = 1 + 1 + 2 + 4 = 8
Product of the digits = 1*1*2*4 = 8A computerised bus charges fare from each of its passengers based on the distance travelled as per the tariff given below:
Distance (in km) Charges First 5 km ₹80 Next 10 km ₹10/km More than 15 km ₹8/km As the passenger enters the bus, the computer prompts 'Enter distance you intend to travel'. On entering the distance, it prints his ticket and the control goes back for the next passenger. At the end of journey, the computer prints the following:
- the number of passenger travelled
- total fare received
Write a program to perform the above task.
[Hint: Perform the task based on user controlled loop]