Computer Applications
Write a menu driven program to accept a number from the user and check whether it is a Prime number or an Automorphic number.
(a) Prime number: (A number is said to be prime, if it is only divisible by 1 and itself)
Example: 3,5,7,11
(b) Automorphic number: (Automorphic number is the number which is contained in the last digit(s) of its square.)
Example: 25 is an Automorphic number as its square is 625 and 25 is present as the last two digits.
Java
Java Iterative Stmts
ICSE 2010
265 Likes
Answer
import java.util.Scanner;
public class KboatPrimeAutomorphic
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Prime number");
System.out.println("2. Automorphic number");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
System.out.print("Enter number: ");
int num = in.nextInt();
switch (choice) {
case 1:
int c = 0;
for (int i = 1; i <= num; i++) {
if (num % i == 0) {
c++;
}
}
if (c == 2)
System.out.println(num + " is Prime");
else
System.out.println(num + " is not Prime");
break;
case 2:
int numCopy = num;
int sq = num * num;
int d = 0;
/*
* Count the number of
* digits in num
*/
while(num > 0) {
d++;
num /= 10;
}
/*
* Extract the last d digits
* from square of num
*/
int ld = (int)(sq % Math.pow(10, d));
if (ld == numCopy)
System.out.println(numCopy + " is automorphic");
else
System.out.println(numCopy + " is not automorphic");
break;
default:
System.out.println("Incorrect Choice");
break;
}
}
}
Variable Description Table
Program Explanation
Output
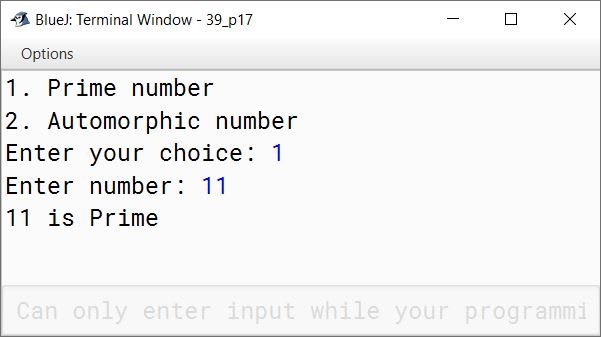
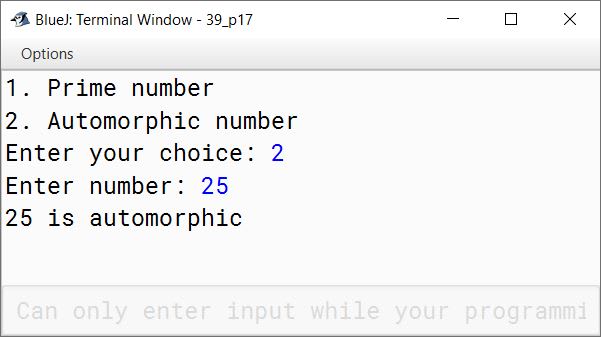
Answered By
87 Likes
Related Questions
Write a menu driven program to input two positive numbers m and n (where m>n) and perform the following tasks:
(a) Find the sum of two numbers without using '+' operator.
(b) Find the product of two numbers without using '*' operator.
(c) Find the quotient and remainder of two numbers without using '/' and '%' operator.
[Hint: The last value obtained after each subtraction is the remainder and the number of iterations results in quotient.]
Sample Input: m=5, n=2
5 - 2 =3
3 - 2 = 1, thus Quotient = 2 and Remainder = 1Write a menu driven class to accept a number from the user and check whether it is a Palindrome or a Perfect number.
(a) Palindrome number: (A number is a Palindrome which when read in reverse order is same as in the right order)
Example: 11, 101, 151 etc.
(b) Perfect number: (A number is called Perfect if it is equal to the sum of its factors other than the number itself.)
Example: 6 = 1 + 2 + 3
Write a menu driven program to perform the following tasks by using Switch case statement:
(a) To print the series:
0, 3, 8, 15, 24, ………… to n terms. (value of 'n' is to be an input by the user)
(b) To find the sum of the series:
S = (1/2) + (3/4) + (5/6) + (7/8) + ……….. + (19/20)
Using a switch statement, write a menu driven program to:
(a) Generate and display the first 10 terms of the Fibonacci series
0, 1, 1, 2, 3, 5
The first two Fibonacci numbers are 0 and 1, and each subsequent number is the sum of the previous two.
(b) Find the sum of the digits of an integer that is input.
Sample Input: 15390
Sample Output: Sum of the digits = 18For an incorrect choice, an appropriate error message should be displayed.