Computer Applications
Write a Java program to print the name of the day of the week using the switch case statement.
Sample Input:
Enter the day number: 1
Sample Output:
Sunday
Java
Java Conditional Stmts
17 Likes
Answer
import java.util.Scanner;
public class KboatDayOfWeek
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the day number: ");
int n = in.nextInt();
switch (n) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
case 3:
System.out.println("Tuesday");
break;
case 4:
System.out.println("Wednesday");
break;
case 5:
System.out.println("Thursday");
break;
case 6:
System.out.println("Friday");
break;
case 7:
System.out.println("Saturday");
break;
default:
System.out.println("Incorrect day.");
}
}
}
Output
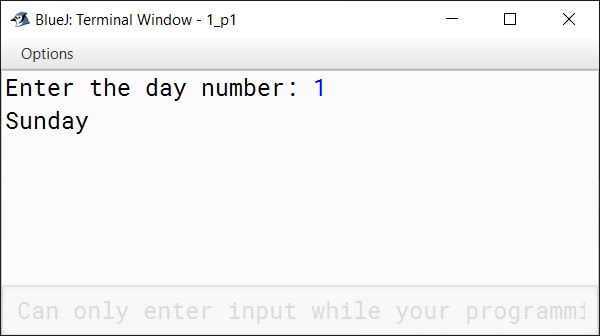
Answered By
6 Likes
Related Questions
Write a Java program to print name, purchase amount and final payable amount after discount as per given table:
Purchase Amount Discount upto ₹10000/- 15% ₹10000 to ₹ 20000/- 20% Above ₹20000/- 30% Write a program using switch case to find the volume of a cube, a sphere and a cuboid.
For an incorrect choice, an appropriate error message should be displayed.- Volume of a cube = s * s *s
- Volume of a sphere = (4/3) * π * r * r * r (π = (22/7))
- Volume of a cuboid = l*b*h
Using the switch-case statement, write a menu driven program to do the following:
(a) To generate and print Letters from A to Z and their Unicode
Letters Unicode A 65 B 66 . . . . . . Z 90 (b) Display the following pattern using iteration (looping) statement:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5A triangle is said to be an 'Equable Triangle', if the area of the triangle is equal to its perimeter. Write a program to enter three sides of a triangle. Check and print whether the triangle is equable or not.
For example, a right angled triangle with sides 5, 12 and 13 has its area and perimeter both equal to 30.