Computer Applications
Write a Java program to print name, purchase amount and final payable amount after discount as per given table:
Purchase Amount | Discount |
---|---|
upto ₹10000/- | 15% |
₹10000 to ₹ 20000/- | 20% |
Above ₹20000/- | 30% |
Java
Java Conditional Stmts
38 Likes
Answer
import java.util.Scanner;
public class KboatDiscount
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Name: ");
String name = in.nextLine();
System.out.print("Enter Purchase Amount: ");
double amt = in.nextInt();
int d = 0;
if (amt <= 10000)
d = 15;
else if (amt <= 20000)
d = 20;
else
d = 30;
double discAmt = amt * d / 100.0;
double finalAmt = amt - discAmt;
System.out.println("Name: " + name);
System.out.println("Purchase Amount: " + amt);
System.out.println("Final Payable Amount: " + finalAmt);
}
}
Output
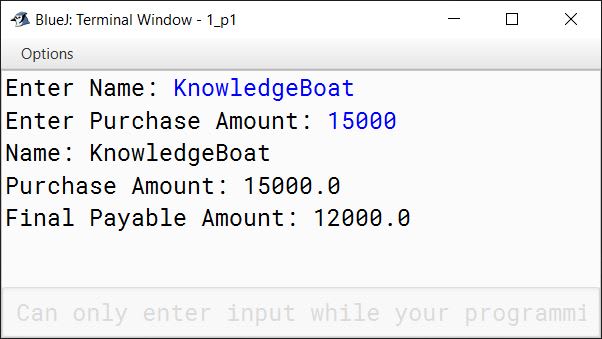
Answered By
12 Likes
Related Questions
Ravi runs the following Java code but encounters an error. Identify the statement causing the issue, correct it, and ensure the output is "Hungry".
char h = 'Y'; if (h == 'y' || 'Y') System.out.println("Hungry"); else System.out.println("Not Hungry");
A triangle is said to be an 'Equable Triangle', if the area of the triangle is equal to its perimeter. Write a program to enter three sides of a triangle. Check and print whether the triangle is equable or not.
For example, a right angled triangle with sides 5, 12 and 13 has its area and perimeter both equal to 30.Which of the following is true about the
default
label in aswitch
statement?Write a program using switch case to find the volume of a cube, a sphere and a cuboid.
For an incorrect choice, an appropriate error message should be displayed.- Volume of a cube = s * s *s
- Volume of a sphere = (4/3) * π * r * r * r (π = (22/7))
- Volume of a cuboid = l*b*h