Computer Applications
Write a class with the name Perimeter using method overloading that computes the perimeter of a square, a rectangle and a circle.
Formula:
Perimeter of a square = 4 * s
Perimeter of a rectangle = 2 * (l + b)
Perimeter of a circle = 2 * (22/7) * r
Java
User Defined Methods
75 Likes
Answer
import java.util.Scanner;
public class Perimeter
{
public double perimeter(double s) {
double p = 4 * s;
return p;
}
public double perimeter(double l, double b) {
double p = 2 * (l + b);
return p;
}
public double perimeter(int c, double pi, double r) {
double p = c * pi * r;
return p;
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
Perimeter obj = new Perimeter();
System.out.print("Enter side of square: ");
double side = in.nextDouble();
System.out.println("Perimeter of square = " + obj.perimeter(side));
System.out.print("Enter length of rectangle: ");
double l = in.nextDouble();
System.out.print("Enter breadth of rectangle: ");
double b = in.nextDouble();
System.out.println("Perimeter of rectangle = " + obj.perimeter(l, b));
System.out.print("Enter radius of circle: ");
double r = in.nextDouble();
System.out.println("Perimeter of circle = " + obj.perimeter(2, 3.14159, r));
}
}
Variable Description Table
Program Explanation
Output
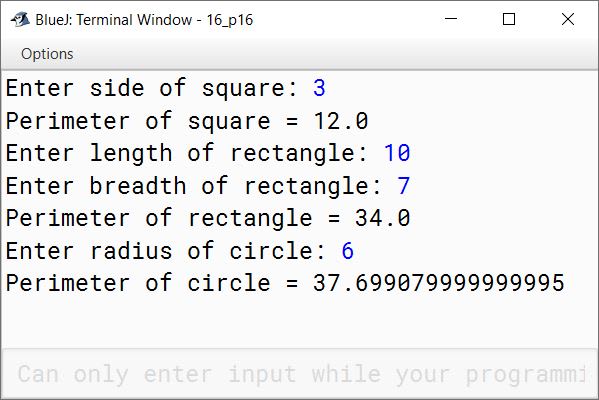
Answered By
27 Likes
Related Questions
Write a class with the name Area using method overloading that computes the area of a parallelogram, a rhombus and a trapezium.
Formula:
Area of a parallelogram (pg) = base * ht
Area of a rhombus (rh) = (1/2) * d1 * d2
(where, d1 and d2 are the diagonals)Area of a trapezium (tr) = (1/2) * ( a + b) * h
(where a and b are the parallel sides, h is the perpendicular distance between the parallel sides)Write a program to accept 10 numbers in a Single Dimensional Array. Pass the array to a method Search(int m[], int ns) to search the given number ns in the list of array elements. If the number is present, then display the message 'Number is present' otherwise, display 'number is not present'.
Design a class overloading and a method display( ) as follows:
- void display(String str, int p) with one String argument and one integer argument. It displays all the uppercase characters if 'p' is 1 (one) otherwise, it displays all the lowercase characters.
- void display(String str, char chr) with one String argument and one character argument. It displays all the vowels if chr is 'v' otherwise, it displays all the alphabets.
Design a class overloading a method calculate() as follows:
- void calculate(int m, char ch) with one integer argument and one character argument. It checks whether the integer argument is divisible by 7 or not, if ch is 's', otherwise, it checks whether the last digit of the integer argument is 7 or not.
- void calculate(int a, int b, char ch) with two integer arguments and one character argument. It displays the greater of integer arguments if ch is 'g' otherwise, it displays the smaller of integer arguments.