Computer Applications
Write a class with the name Area using method overloading that computes the area of a parallelogram, a rhombus and a trapezium.
Formula:
Area of a parallelogram (pg) = base * ht
Area of a rhombus (rh) = (1/2) * d1 * d2
(where, d1 and d2 are the diagonals)
Area of a trapezium (tr) = (1/2) * ( a + b) * h
(where a and b are the parallel sides, h is the perpendicular distance between the parallel sides)
Java
User Defined Methods
80 Likes
Answer
import java.util.Scanner;
public class Area
{
public double area(double base, double height) {
double a = base * height;
return a;
}
public double area(double c, double d1, double d2) {
double a = c * d1 * d2;
return a;
}
public double area(double c, double a, double b, double h) {
double x = c * (a + b) * h;
return x;
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
Area obj = new Area();
System.out.print("Enter base of parallelogram: ");
double base = in.nextDouble();
System.out.print("Enter height of parallelogram: ");
double ht = in.nextDouble();
System.out.println("Area of parallelogram = " + obj.area(base, ht));
System.out.print("Enter first diagonal of rhombus: ");
double d1 = in.nextDouble();
System.out.print("Enter second diagonal of rhombus: ");
double d2 = in.nextDouble();
System.out.println("Area of rhombus = " + obj.area(0.5, d1, d2));
System.out.print("Enter first parallel side of trapezium: ");
double a = in.nextDouble();
System.out.print("Enter second parallel side of trapezium: ");
double b = in.nextDouble();
System.out.print("Enter height of trapezium: ");
double h = in.nextDouble();
System.out.println("Area of trapezium = " + obj.area(0.5, a, b, h));
}
}
Variable Description Table
Program Explanation
Output
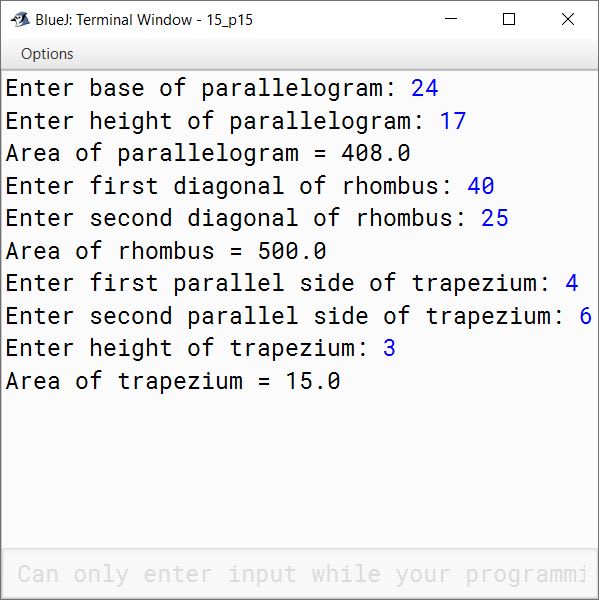
Answered By
33 Likes
Related Questions
Write a program to accept 10 numbers in a Single Dimensional Array. Pass the array to a method Search(int m[], int ns) to search the given number ns in the list of array elements. If the number is present, then display the message 'Number is present' otherwise, display 'number is not present'.
Write a class with the name Perimeter using method overloading that computes the perimeter of a square, a rectangle and a circle.
Formula:
Perimeter of a square = 4 * s
Perimeter of a rectangle = 2 * (l + b)
Perimeter of a circle = 2 * (22/7) * r
Design a class overloading and a method display( ) as follows:
- void display(String str, int p) with one String argument and one integer argument. It displays all the uppercase characters if 'p' is 1 (one) otherwise, it displays all the lowercase characters.
- void display(String str, char chr) with one String argument and one character argument. It displays all the vowels if chr is 'v' otherwise, it displays all the alphabets.
Write a program in Java to accept a String from the user. Pass the String to a method First(String str) which displays the first character of each word.
Sample Input : Understanding Computer Applications
Sample Output:
U
C
A