Computer Applications
Design a class overloading and a method display( ) as follows:
- void display(String str, int p) with one String argument and one integer argument. It displays all the uppercase characters if 'p' is 1 (one) otherwise, it displays all the lowercase characters.
- void display(String str, char chr) with one String argument and one character argument. It displays all the vowels if chr is 'v' otherwise, it displays all the alphabets.
Java
User Defined Methods
51 Likes
Answer
import java.util.Scanner;
public class Overloading
{
void display(String str, int p) {
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (p == 1 && Character.isUpperCase(ch)) {
System.out.println(ch);
}
else if (p != 1 && Character.isLowerCase(ch)) {
System.out.println(ch);
}
}
}
void display(String str, char chr) {
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
ch = Character.toUpperCase(ch);
if (chr != 'v' && Character.isLetter(str.charAt(i)))
System.out.println(str.charAt(i));
else if (ch == 'A' ||
ch == 'E' ||
ch == 'I' ||
ch == 'O' ||
ch == 'U') {
System.out.println(str.charAt(i));
}
}
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter string: ");
String s = in.nextLine();
Overloading obj = new Overloading();
System.out.println("p=1");
obj.display(s, 1);
System.out.println("\np!=1");
obj.display(s, 0);
System.out.println("\nchr='v'");
obj.display(s, 'v');
System.out.println("\nchr!='v'");
obj.display(s, 'u');
}
}
Variable Description Table
Program Explanation
Output
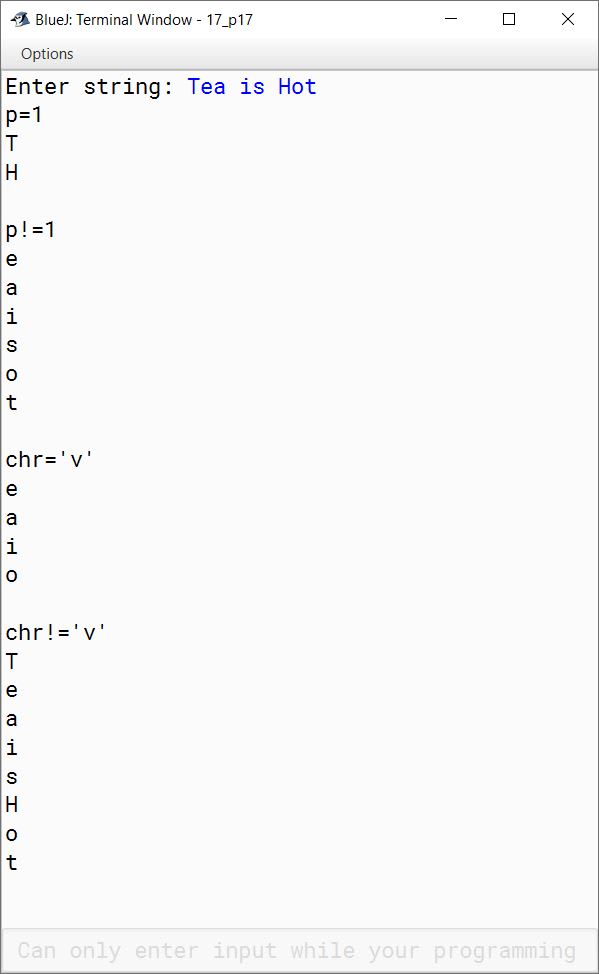
Answered By
17 Likes
Related Questions
Write a class with the name Area using method overloading that computes the area of a parallelogram, a rhombus and a trapezium.
Formula:
Area of a parallelogram (pg) = base * ht
Area of a rhombus (rh) = (1/2) * d1 * d2
(where, d1 and d2 are the diagonals)Area of a trapezium (tr) = (1/2) * ( a + b) * h
(where a and b are the parallel sides, h is the perpendicular distance between the parallel sides)Design a class to overload a method compare( ) as follows:
- void compare(int, int) — to compare two integers values and print the greater of the two integers.
- void compare(char, char) — to compare the numeric value of two characters and print with the higher numeric value.
- void compare(String, String) — to compare the length of the two strings and print the longer of the two.
Write a class with the name Perimeter using method overloading that computes the perimeter of a square, a rectangle and a circle.
Formula:
Perimeter of a square = 4 * s
Perimeter of a rectangle = 2 * (l + b)
Perimeter of a circle = 2 * (22/7) * r
Design a class overloading a method calculate() as follows:
- void calculate(int m, char ch) with one integer argument and one character argument. It checks whether the integer argument is divisible by 7 or not, if ch is 's', otherwise, it checks whether the last digit of the integer argument is 7 or not.
- void calculate(int a, int b, char ch) with two integer arguments and one character argument. It displays the greater of integer arguments if ch is 'g' otherwise, it displays the smaller of integer arguments.