Computer Science
Input a paragraph containing 'n' number of sentences where (1 < = n < 4). The words are to be separated with a single blank space and are in UPPERCASE. A sentence may be terminated either with a full stop '.' Or a question mark '?' only. Any other character may be ignored. Perform the following operations:
- Accept the number of sentences. If the number of sentences exceeds the limit, an appropriate error message must be displayed.
- Find the number of words in the whole paragraph.
- Display the words in ascending order of their frequency. Words with same frequency may appear in any order.
Example 1
Input:
Enter number of sentences.
1
Enter sentences.
TO BE OR NOT TO BE.
Output:
Total number of words: 6
Word | Frequency |
---|---|
OR | 1 |
NOT | 1 |
TO | 2 |
BE | 2 |
Example 2
Input:
Enter number of sentences.
3
Enter sentences.
THIS IS A STRING PROGRAM. IS THIS EASY? YES IT IS.
Output:
Total number of words: 11
Word | Frequency |
---|---|
A | 1 |
STRING | 1 |
PROGRAM | 1 |
EASY | 1 |
YES | 1 |
IT | 1 |
THIS | 2 |
IS | 3 |
Example 3
Input:
Enter number of sentences. 5
Output:
Invalid Entry
Java
Java String Handling
6 Likes
Answer
import java.util.*;
public class KboatPara
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter number of sentences.");
int n = in.nextInt();
in.nextLine();
if (n < 1 || n > 3) {
System.out.println("Invalid Entry");
return;
}
System.out.println("Enter sentences.");
String ipStr = in.nextLine();
ipStr = ipStr.toUpperCase();
StringTokenizer st = new StringTokenizer(ipStr, " .?");
int wordCount = st.countTokens();
System.out.println();
System.out.println("Total number of words: " + wordCount);
String wordArr[] = new String[wordCount];
int wordFreq[] = new int[wordCount];
int idx = 0;
for (int i = 0; i < wordCount; i++) {
String word = st.nextToken();
int j = 0;
for (j = 0; j < idx; j++) {
if (wordArr[j].equals(word)) {
wordFreq[j]++;
break;
}
}
if (j == idx) {
wordArr[idx] = word;
wordFreq[idx]++;
idx++;
}
}
//Sort Word Frequency in ascending order
for (int i = 0; i < idx - 1; i++) {
for (int j = 0; j < idx - i - 1; j++) {
if (wordFreq[j] > wordFreq[j + 1]) {
int t = wordFreq[j];
wordFreq[j] = wordFreq[j+1];
wordFreq[j+1] = t;
String temp = wordArr[j];
wordArr[j] = wordArr[j+1];
wordArr[j+1] = temp;
}
}
}
//Display the words and frequencies
System.out.println("Word\tFrequency");
for (int i = 0; i < idx; i++) {
System.out.println(wordArr[i] + "\t" + wordFreq[i]);
}
}
}
Output
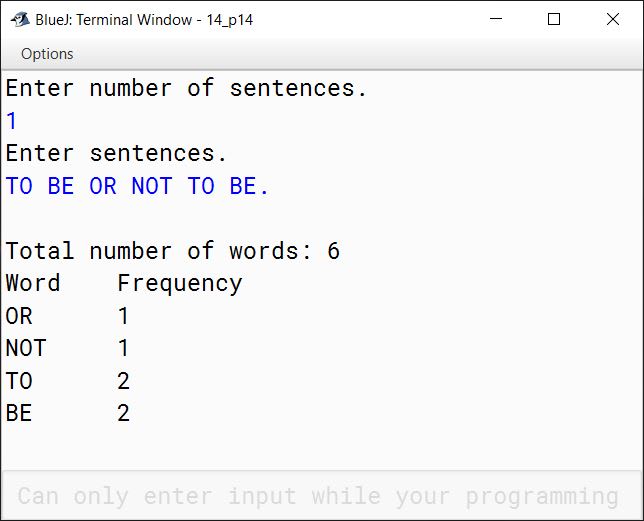
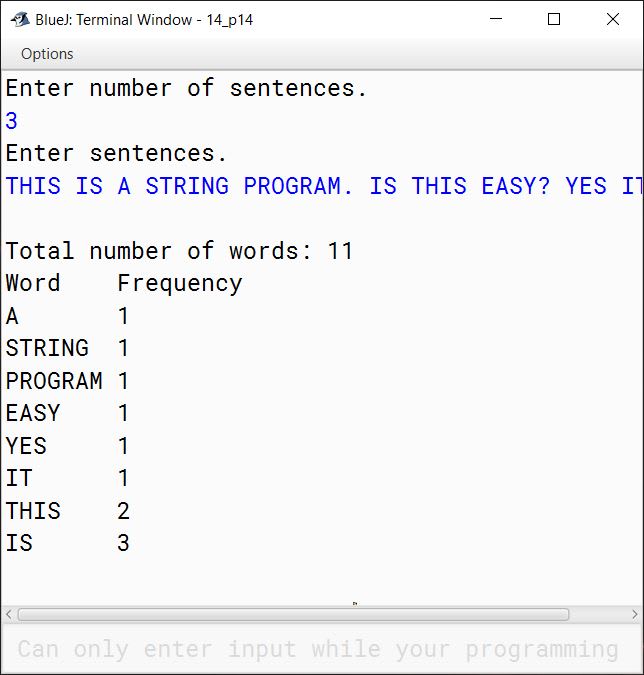
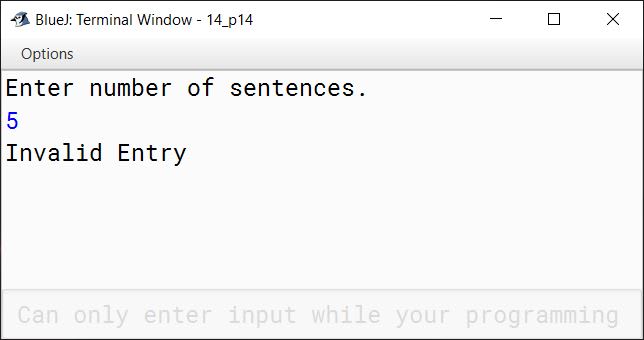
Answered By
1 Like
Related Questions
Read a single sentence which terminates with a full stop (.). The words are to be separated with a single blank space and are in lower case. Arrange the words contained in the sentence according to the length of the words in ascending order. If two words are of the same length then the word occurring first in the input sentence should come first. For both, input and output the sentence must begin in upper case.
Test your program for given data and also some random data:
Input:
The lines are printed in reverse order.
Output:
In the are lines order printed reverse.Input:
Print the sentence in ascending order.
Output:
In the print order sentence ascending.Input:
I love my Country.
Output:
I my love Country.Write a program to read a string / sentence and output the number of times each word occurs in the entire text. At the end, the output should be sorted into ascending order words along with usage of words. You may assume that the entire text is in capitals (you may also convert in capitals for your betterment) and each word is followed by a blank space except the last one, which is followed by a full stop. Let there be at the most 50 words in the text.
Test your program for the given sample data and also some other random data:
SAMPLE DATA:
Input:
YOU ARE GOOD WHEN YOUR THOUGHTS ARE GOOD AND YOUR DEEDS ARE GOOD.Output:
Words Word Count And 1 Are 3 Deeds 1 Good 3 Thoughts 1 When 1 You 1 Your 2 Input:
IF YOU FAIL TO PLAN YOU ARE PLANNING TO FAIL.Output:
Words Word Count Are 1 Fail 2 If 1 Plan 1 Planning 1 To 2 You 2 A string is said to be valid if it contains pair of parenthesis having text / alphabet such as (TY) and the string is said to be invalid if it contains nested parenthesis such as (Y (UP)).
For example:
SUN (A(X) RISE) BEGINS FROM (RT) EAST is an "Invalid" string because in this string nested parenthesis are present, but SUN (A) RISE BEGINS FROM (RT) EAST is a "Valid" string because there is no nested parenthesis.Write a program to:
- Read a string / sentence and convert in capitals.
- Check the validity of the given string.
- If the string is valid, output the given string omitting the portion enclosed in brackets otherwise, output a message "Sorry, and invalid string".
Test your program for the given sample data and also some other random data:
Sample Data:
Input:
SUN (a) RISE BEGINS FROM (RT) EAST
Output:
SUN RISE BEGINS FROM EASTInput:
SUN (A (X) RISE) BEGINS FROM (RT) EAST
Output:
Sorry, an invalid stringInput:
COM(IPX)PUTER IS (MY) JUNK (GOOD) SYSTEM
Output:
COMPUTER IS JUNK SYSTEMCaesar Cipher is an encryption technique which is implemented as ROT13 ('rotate by 13 places'). It is a simple letter substitution cipher that replaces a letter with the letter 13 places after it in the alphabets, with the other characters remaining unchanged.
ROT13
A/a B/b C/c D/d E/e F/f G/g H/h I/i J/j K/k L/l M/m ↕ ↕ ↕ ↕ ↕ ↕ ↕ ↕ ↕ ↕ ↕ ↕ ↕ N/n O/o P/p Q/q R/r S/s T/t U/u V/v W/w X/x Y/y Z/z Write a program to accept a plain text of length L, where L must be greater than 3 and less than 100.
Encrypt the text if valid as per the Caesar Cipher.
Test your program with the sample data and some random data.
Example 1
INPUT:
Hello! How are you?OUTPUT:
The cipher text is:
Uryyb! Ubj ner lbh?Example 2
INPUT:
Encryption helps to secure data.OUTPUT:
The cipher text is:
Rapelcgvba urycf gb frpher qngn.Example 3
INPUT:
YouOUTPUT:
INVALID LENGTH