Computer Science
Caesar Cipher is an encryption technique which is implemented as ROT13 ('rotate by 13 places'). It is a simple letter substitution cipher that replaces a letter with the letter 13 places after it in the alphabets, with the other characters remaining unchanged.
ROT13
A/a | B/b | C/c | D/d | E/e | F/f | G/g | H/h | I/i | J/j | K/k | L/l | M/m |
↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ | ↕ |
N/n | O/o | P/p | Q/q | R/r | S/s | T/t | U/u | V/v | W/w | X/x | Y/y | Z/z |
Write a program to accept a plain text of length L, where L must be greater than 3 and less than 100.
Encrypt the text if valid as per the Caesar Cipher.
Test your program with the sample data and some random data.
Example 1
INPUT:
Hello! How are you?
OUTPUT:
The cipher text is:
Uryyb! Ubj ner lbh?
Example 2
INPUT:
Encryption helps to secure data.
OUTPUT:
The cipher text is:
Rapelcgvba urycf gb frpher qngn.
Example 3
INPUT:
You
OUTPUT:
INVALID LENGTH
Java
Java String Handling
ICSE Prac 2017
16 Likes
Answer
import java.util.Scanner;
public class CaesarCipher
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter plain text:");
String str = in.nextLine();
int len = str.length();
if (len <= 3 || len >= 100) {
System.out.println("INVALID LENGTH");
return;
}
StringBuffer sb = new StringBuffer();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if ((ch >= 'A' && ch <= 'M') || (ch >= 'a' && ch <= 'm')) {
sb.append((char)(ch + 13));
}
else if ((ch >= 'N' && ch <= 'Z') || (ch >= 'n' && ch <= 'z')) {
sb.append((char)(ch - 13));
}
else {
sb.append(ch);
}
}
String cipher = sb.toString();
System.out.println("The cipher text is:");
System.out.println(cipher);
}
}
Output
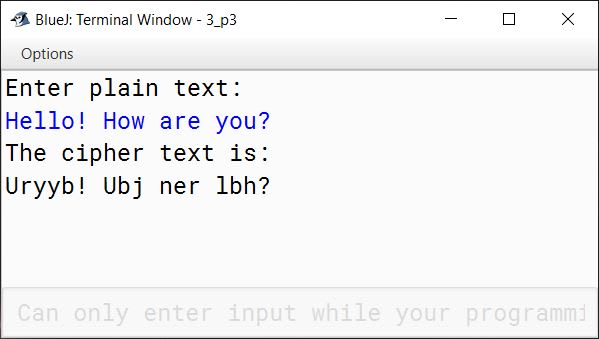
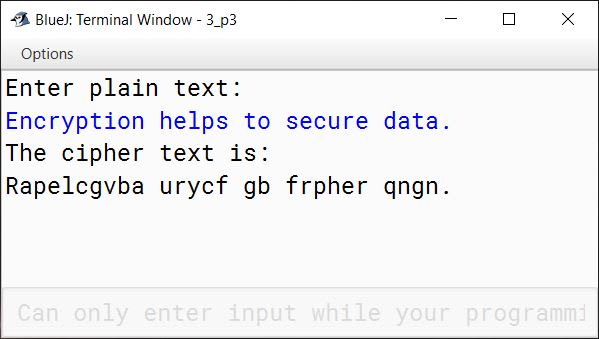
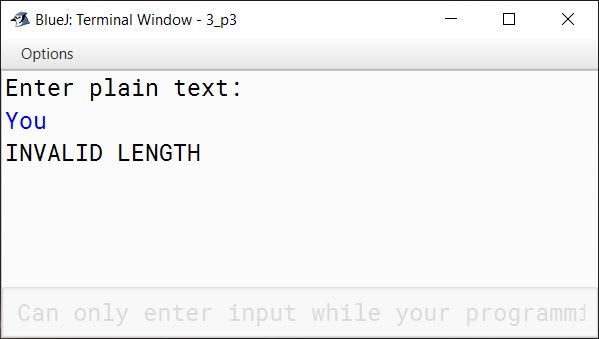
Answered By
7 Likes
Related Questions
Two strings,
city1
andcity2
, are compared usingcity1.compareTo(city2)
, and the result is less than zero. What does this indicate?Define a class to accept a string and convert the same to uppercase, create and display the new string by replacing each vowel by immediate next character and every consonant by the previous character. The other characters remain the same.
Example:
Input : #IMAGINATION@2024
Output : #JLBFJMBSJPM@2024A shopping website offers a special discount if the order ID has the sequence 555 anywhere in it. For example, 158545553031, 198555267140, …. .
Fill in the blanks (a) and (b) in the given Java Method to convert the order ID (a long integer) into a string and check if the sequence 555 is present in it.
void checkOrder(long oid) { String str = _______(a)_________; if(______(b)_______) { System.out.println("Special Discount Eligible: " + oid); } }
Define a class to accept the gmail id and check for its validity.
A gmail id is valid only if it has:
→ @
→ .(dot)
→ gmail
→ com
Example:
icse2024@gmail.com
is a valid gmail id