Computer Science
Write a program to read a string / sentence and output the number of times each word occurs in the entire text. At the end, the output should be sorted into ascending order words along with usage of words. You may assume that the entire text is in capitals (you may also convert in capitals for your betterment) and each word is followed by a blank space except the last one, which is followed by a full stop. Let there be at the most 50 words in the text.
Test your program for the given sample data and also some other random data:
SAMPLE DATA:
Input:
YOU ARE GOOD WHEN YOUR THOUGHTS ARE GOOD AND YOUR DEEDS ARE GOOD.
Output:
Words | Word Count |
---|---|
And | 1 |
Are | 3 |
Deeds | 1 |
Good | 3 |
Thoughts | 1 |
When | 1 |
You | 1 |
Your | 2 |
Input:
IF YOU FAIL TO PLAN YOU ARE PLANNING TO FAIL.
Output:
Words | Word Count |
---|---|
Are | 1 |
Fail | 2 |
If | 1 |
Plan | 1 |
Planning | 1 |
To | 2 |
You | 2 |
Java
Java String Handling
9 Likes
Answer
import java.util.*;
public class KboatWordFreq
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a string:");
String str = in.nextLine();
str = str.toUpperCase();
int len = str.length();
StringTokenizer st = new StringTokenizer(str.substring(0, len - 1));
int wordCount = st.countTokens();
String strArr[] = new String[wordCount];
if (wordCount > 50) {
System.out.println("Invalid Input!");
System.out.println("Number of words should be upto 50 only.");
return;
}
for (int i = 0; i < wordCount; i++) {
strArr[i] = st.nextToken();
}
for (int i = 0; i < wordCount - 1; i++) {
for (int j = 0; j < wordCount - i - 1; j++) {
if (strArr[j].compareTo(strArr[j+1]) > 0) {
String t = strArr[j];
strArr[j] = strArr[j+1];
strArr[j+1] = t;
}
}
}
System.out.println(); //To Print a blank line
System.out.println("Word\t\tWord Count");
int count = 0;
for (int i = 0; i < wordCount - 1; i++) {
count++;
if (!strArr[i].equals(strArr[i + 1])) {
System.out.println(strArr[i] + "\t\t" + count);
count = 0;
}
}
//Print last word of array
count++;
System.out.println(strArr[wordCount - 1] + "\t\t" + count);
}
}
Output
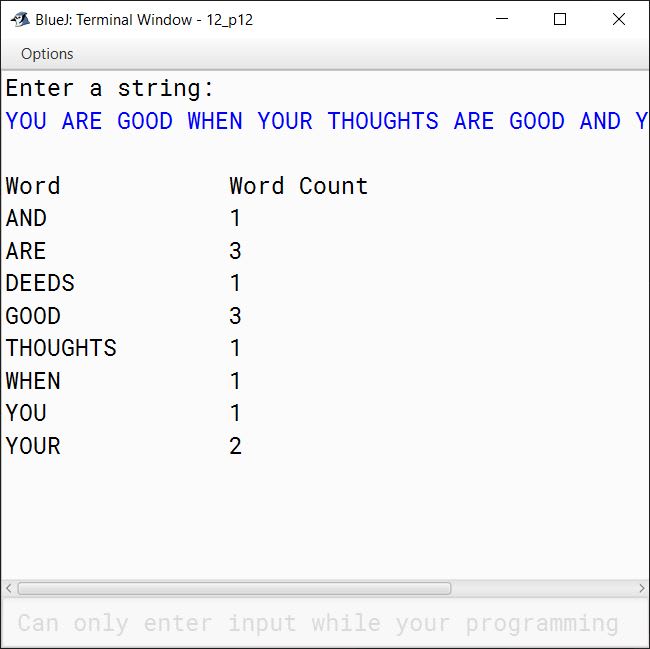
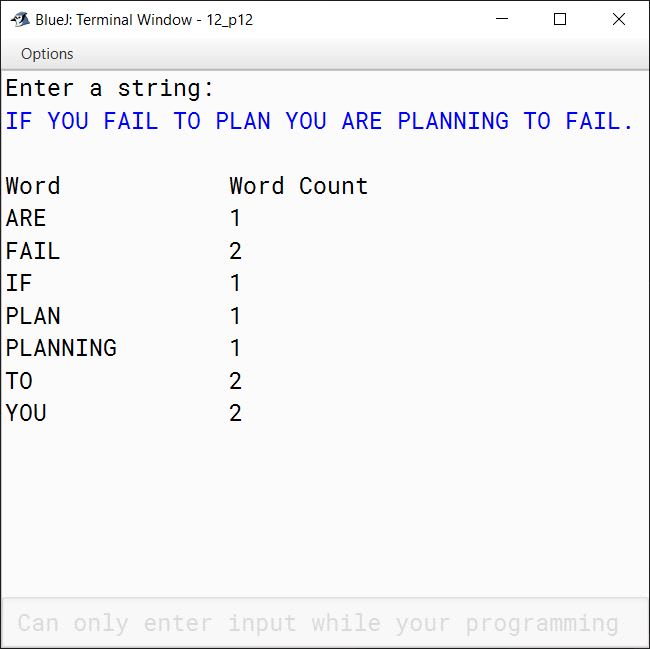
Answered By
5 Likes
Related Questions
The input in this question will consist of a number of lines of English text consisting of the letters of the English alphabet, the punctuation marks (') apostrophe, (.) full stop (, ) comma, (; ) semicolon, (:) colon and white space characters (blank, new line). Your task is to print the words of the text in reverse order without any punctuation marks other than blanks.
For example, consider the following input text:
This is a sample piece of text to illustrate this question.
If you are smart you will solve this right.The corresponding output would read as:
right this solve will you smart are you if question this illustrate to text of piece sample a is this
Note: Individual words are not reversed.
Input Format:
This first line of input contains a single integer n ( < = 20), indicating the number of lines in the input.
This is followed by lines of input text. Each line should accept a maximum of 80 characters.Output Format:
Output the text containing the input lines in reverse order without punctuations except blanks as illustrated above.
Test your program for the following data and some random data.
Sample Data:
Input:
2
Emotions controlled and directed to work, is character.
By Swami Vivekananda.Output:
Vivekananda Swami By character is work to directed and controlled EmotionsInput:
1
Do not judge a book by its cover.Output:
cover its by book a judge not DoRead a single sentence which terminates with a full stop (.). The words are to be separated with a single blank space and are in lower case. Arrange the words contained in the sentence according to the length of the words in ascending order. If two words are of the same length then the word occurring first in the input sentence should come first. For both, input and output the sentence must begin in upper case.
Test your program for given data and also some random data:
Input:
The lines are printed in reverse order.
Output:
In the are lines order printed reverse.Input:
Print the sentence in ascending order.
Output:
In the print order sentence ascending.Input:
I love my Country.
Output:
I my love Country.A string is said to be valid if it contains pair of parenthesis having text / alphabet such as (TY) and the string is said to be invalid if it contains nested parenthesis such as (Y (UP)).
For example:
SUN (A(X) RISE) BEGINS FROM (RT) EAST is an "Invalid" string because in this string nested parenthesis are present, but SUN (A) RISE BEGINS FROM (RT) EAST is a "Valid" string because there is no nested parenthesis.Write a program to:
- Read a string / sentence and convert in capitals.
- Check the validity of the given string.
- If the string is valid, output the given string omitting the portion enclosed in brackets otherwise, output a message "Sorry, and invalid string".
Test your program for the given sample data and also some other random data:
Sample Data:
Input:
SUN (a) RISE BEGINS FROM (RT) EAST
Output:
SUN RISE BEGINS FROM EASTInput:
SUN (A (X) RISE) BEGINS FROM (RT) EAST
Output:
Sorry, an invalid stringInput:
COM(IPX)PUTER IS (MY) JUNK (GOOD) SYSTEM
Output:
COMPUTER IS JUNK SYSTEMInput a paragraph containing 'n' number of sentences where (1 < = n < 4). The words are to be separated with a single blank space and are in UPPERCASE. A sentence may be terminated either with a full stop '.' Or a question mark '?' only. Any other character may be ignored. Perform the following operations:
- Accept the number of sentences. If the number of sentences exceeds the limit, an appropriate error message must be displayed.
- Find the number of words in the whole paragraph.
- Display the words in ascending order of their frequency. Words with same frequency may appear in any order.
Example 1
Input:
Enter number of sentences.
1
Enter sentences.
TO BE OR NOT TO BE.Output:
Total number of words: 6Word Frequency OR 1 NOT 1 TO 2 BE 2 Example 2
Input:
Enter number of sentences.
3
Enter sentences.
THIS IS A STRING PROGRAM. IS THIS EASY? YES IT IS.Output:
Total number of words: 11Word Frequency A 1 STRING 1 PROGRAM 1 EASY 1 YES 1 IT 1 THIS 2 IS 3 Example 3
Input:
Enter number of sentences. 5Output:
Invalid Entry