Computer Applications
Define a class to search for a value input by the user from the list of values given below. If it is found display the message "Search successful", otherwise display the message "Search element not found" using Binary search technique.
5.6, 11.5, 20.8, 35.4, 43.1, 52.4, 66.6, 78.9, 80.0, 95.5.
Java
Java Arrays
ICSE Sp 2025
10 Likes
Answer
import java.util.Scanner;
public class KboatBinarySearch
{
void search(double[] arr, double key) {
int low = 0;
int high = arr.length - 1;
boolean found = false;
while (low <= high) {
int mid = (low + high) / 2;
if (arr[mid] == key) {
found = true;
break;
} else if (arr[mid] < key) {
low = mid + 1;
} else {
high = mid - 1;
}
}
if (found) {
System.out.println("Search successful");
} else {
System.out.println("Search element not found");
}
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
double[] values = {5.6, 11.5, 20.8, 35.4, 43.1, 52.4, 66.6, 78.9, 80.0, 95.5};
System.out.print("Enter the value to search: ");
double key = in.nextDouble();
KboatBinarySearch obj = new KboatBinarySearch();
obj.search(values, key);
}
}
Variable Description Table
Program Explanation
Output
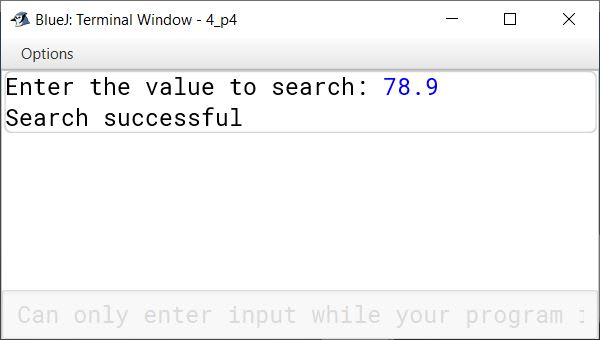
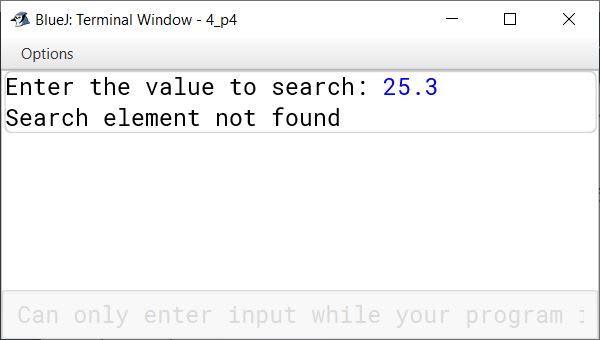
Answered By
5 Likes
Related Questions
Consider the following program segment and answer the questions given below:
int x[][] = {{2,4,5,6}, {5,7,8,1}, {34, 1, 10, 9}};
(a) What is the position of 34?
(b) What is the result of x[2][3] + x[1][2]?
Define a class with the following specifications:
Class name: Bank
Member variables:
double p — stores the principal amount
double n — stores the time period in years
double r — stores the rate of interest
double a — stores the amountMember methods:
void accept () — input values for p and n using Scanner class methods only.
void calculate () — calculate the amount based on the following conditions:Time in (Years) Rate % Upto 1⁄2 9 > 1⁄2 to 1 year 10 > 1 to 3 years 11 > 3 years 12 void display () — display the details in the given format.
Principal Time Rate Amount XXX XXX XXX XXX
Write the main method to create an object and call the above methods.
Define a class to accept a string and convert the same to uppercase, create and display the new string by replacing each vowel by immediate next character and every consonant by the previous character. The other characters remain the same.
Example:
Input : #IMAGINATION@2024
Output : #JLBFJMBSJPM@2024Define a class to accept values into 4x4 array and find and display the sum of each row.
Example:
A[][]={{1,2,3,4},{5,6,7,8},{1,3,5,7},{2,5,3,1}}
Output:
sum of row 1 = 10 (1+2+3+4) sum of row 2 = 26 (5+6+7+8) sum of row 3 = 16 (1+3+5+7) sum of row 4 = 11 (2+5+3+1)