Computer Applications
Define a class with the following specifications:
Class name: Bank
Member variables:
double p — stores the principal amount
double n — stores the time period in years
double r — stores the rate of interest
double a — stores the amount
Member methods:
void accept () — input values for p and n using Scanner class methods only.
void calculate () — calculate the amount based on the following conditions:
Time in (Years) | Rate % |
---|---|
Upto 1⁄2 | 9 |
> 1⁄2 to 1 year | 10 |
> 1 to 3 years | 11 |
> 3 years | 12 |
void display () — display the details in the given format.
Principal Time Rate Amount
XXX XXX XXX XXX
Write the main method to create an object and call the above methods.
Java
Java Classes
ICSE Sp 2025
16 Likes
Answer
import java.util.Scanner;
public class Bank
{
private double p;
private double n;
private double r;
private double a;
void accept() {
Scanner in = new Scanner(System.in);
System.out.print("Enter principal amount: ");
p = in.nextDouble();
System.out.print("Enter time period in years: ");
n = in.nextDouble();
}
void calculate() {
if (n <= 0.5) {
r = 9;
} else if (n <= 1) {
r = 10;
} else if (n <= 3) {
r = 11;
} else {
r = 12;
}
a = p * Math.pow(1 + (r / 100), n);
}
void display() {
System.out.println("Principal\tTime\tRate\tAmount");
System.out.println(p + "\t" + n + "\t" + r + "\t" + a);
}
public static void main(String args[]) {
Bank b = new Bank();
b.accept();
b.calculate();
b.display();
}
}
Variable Description Table
Program Explanation
Output
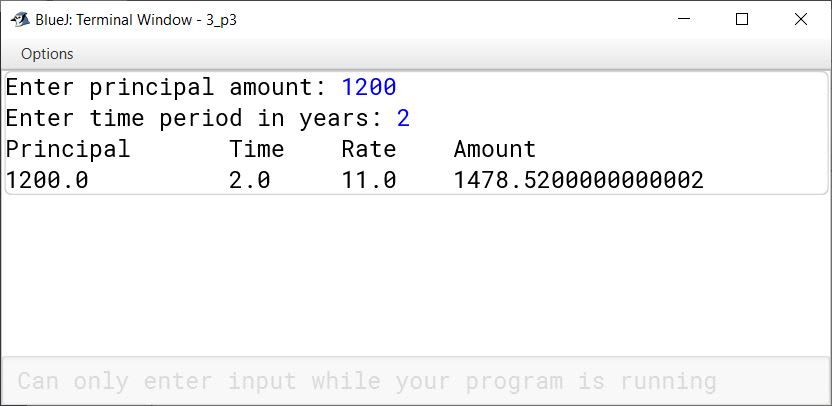
Answered By
10 Likes
Related Questions
Consider the following program segment and answer the questions below:
class calculate { int a; double b; calculate() { a=0; b=0.0; } calculate(int x, double y) { a=x; b=y; } void sum() { System.out.println(a*b); }}
Name the type of constructors used in the above program segment?
Consider the following program segment and answer the questions given below:
int x[][] = {{2,4,5,6}, {5,7,8,1}, {34, 1, 10, 9}};
(a) What is the position of 34?
(b) What is the result of x[2][3] + x[1][2]?
Define a class to search for a value input by the user from the list of values given below. If it is found display the message "Search successful", otherwise display the message "Search element not found" using Binary search technique.
5.6, 11.5, 20.8, 35.4, 43.1, 52.4, 66.6, 78.9, 80.0, 95.5.
Define a class to accept a string and convert the same to uppercase, create and display the new string by replacing each vowel by immediate next character and every consonant by the previous character. The other characters remain the same.
Example:
Input : #IMAGINATION@2024
Output : #JLBFJMBSJPM@2024