Computer Applications
Define a class to accept a string and convert the same to uppercase, create and display the new string by replacing each vowel by immediate next character and every consonant by the previous character. The other characters remain the same.
Example:
Input : #IMAGINATION@2024
Output : #JLBFJMBSJPM@2024
Java
Java String Handling
ICSE Sp 2025
6 Likes
Answer
import java.util.Scanner;
public class KboatStringConvert
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a string: ");
String str = in.nextLine();
str = str.toUpperCase();
int l = str.length();
String res = "";
for (int i = 0; i < l; i++) {
char ch = str.charAt(i);
if ("AEIOU".indexOf(ch) != -1) {
res += (char)(ch + 1);
}
else if (Character.isLetter(ch)) {
res += (char)(ch - 1);
}
else {
res += ch;
}
}
System.out.println("Output String:");
System.out.println(res);
}
}
Variable Description Table
Program Explanation
Output
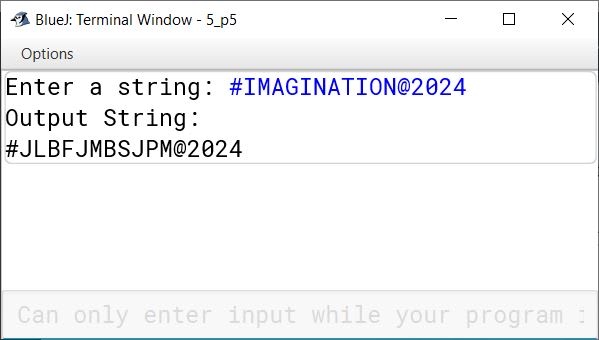
Answered By
3 Likes
Related Questions
Define a class with the following specifications:
Class name: Bank
Member variables:
double p — stores the principal amount
double n — stores the time period in years
double r — stores the rate of interest
double a — stores the amountMember methods:
void accept () — input values for p and n using Scanner class methods only.
void calculate () — calculate the amount based on the following conditions:Time in (Years) Rate % Upto 1⁄2 9 > 1⁄2 to 1 year 10 > 1 to 3 years 11 > 3 years 12 void display () — display the details in the given format.
Principal Time Rate Amount XXX XXX XXX XXX
Write the main method to create an object and call the above methods.
Define a class to search for a value input by the user from the list of values given below. If it is found display the message "Search successful", otherwise display the message "Search element not found" using Binary search technique.
5.6, 11.5, 20.8, 35.4, 43.1, 52.4, 66.6, 78.9, 80.0, 95.5.
Define a class to accept values into 4x4 array and find and display the sum of each row.
Example:
A[][]={{1,2,3,4},{5,6,7,8},{1,3,5,7},{2,5,3,1}}
Output:
sum of row 1 = 10 (1+2+3+4) sum of row 2 = 26 (5+6+7+8) sum of row 3 = 16 (1+3+5+7) sum of row 4 = 11 (2+5+3+1)
Define a class to accept a number and check whether it is a SUPERSPY number or not. A number is called SUPERSPY if the sum of the digits equals the number of the digits.
Example1:
Input: 1021 output: SUPERSPY number [SUM OF THE DIGITS = 1+0+2+1 = 4, NUMBER OF DIGITS = 4 ]
Example2:
Input: 125 output: Not an SUPERSPY number [1+2+5 is not equal to 3]