Computer Applications
Define a class to perform binary search on a list of integers given below, to search for an element input by the user, if it is found display the element along with its position, otherwise display the message "Search element not found".
2, 5, 7, 10, 15, 20, 29, 30, 46, 50
Java
Java Arrays
ICSE 2022
18 Likes
Answer
import java.util.Scanner;
public class KboatBinarySearch
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = {2, 5, 7, 10, 15, 20, 29, 30, 46, 50};
System.out.print("Enter number to search: ");
int n = in.nextInt();
int l = 0, h = arr.length - 1, index = -1;
while (l <= h) {
int m = (l + h) / 2;
if (arr[m] < n)
l = m + 1;
else if (arr[m] > n)
h = m - 1;
else {
index = m;
break;
}
}
if (index == -1) {
System.out.println("Search element not found");
}
else {
System.out.println(n + " found at position " + index);
}
}
}
Variable Description Table
Program Explanation
Output
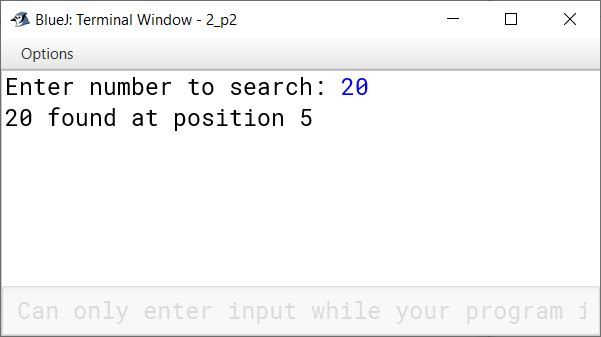
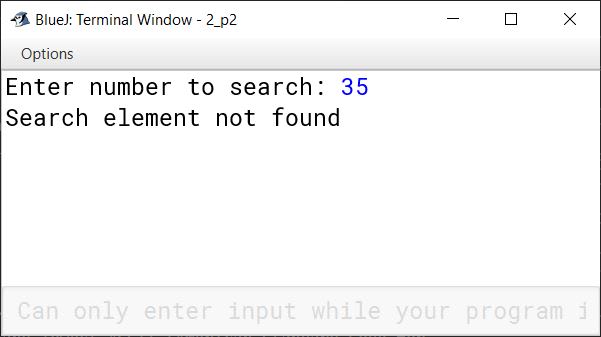
Answered By
7 Likes
Related Questions
Give the output of the following code :
String A = "56.0", B = "94.0"; double C = Double.parseDouble(A); double D = Double.parseDouble(B); System.out.println((C+D));
- 100
- 150.0
- 100.0
- 150
What will be the output of the following code?
System.out.println("Lucknow".substring(0,4));
- Lucknow
- Luckn
- Luck
- luck
Define a class to declare a character array of size ten. Accept the characters into the array and display the characters with highest and lowest ASCII (American Standard Code for Information Interchange) value.
EXAMPLE :
INPUT:
'R', 'z', 'q', 'A', 'N', 'p', 'm', 'U', 'Q', 'F'
OUTPUT :
Character with highest ASCII value = z
Character with lowest ASCII value = ADefine a class to declare an array of size twenty of double datatype, accept the elements into the array and perform the following :
Calculate and print the product of all the elements.
Print the square of each element of the array.