Computer Applications
Define a class to declare an array of size twenty of double datatype, accept the elements into the array and perform the following :
Calculate and print the product of all the elements.
Print the square of each element of the array.
Java
Java Arrays
ICSE 2022
25 Likes
Answer
import java.util.Scanner;
public class KboatSDADouble
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
double arr[] = new double[20];
int l = arr.length;
double p = 1.0;
System.out.println("Enter 20 numbers:");
for (int i = 0; i < l; i++)
{
arr[i] = in.nextDouble();
}
for (int i = 0; i < l; i++)
{
p *= arr[i];
}
System.out.println("Product = " + p);
System.out.println("Square of array elements :");
for (int i = 0; i < l; i++)
{
double sq = Math.pow(arr[i], 2);
System.out.println(sq + " ");
}
}
}
Variable Description Table
Program Explanation
Output
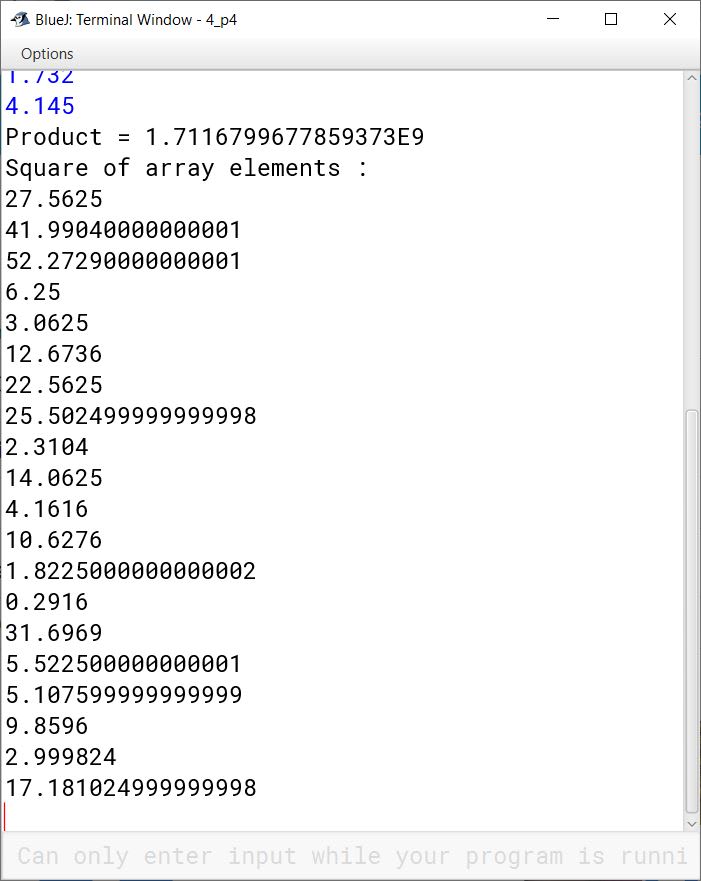
Answered By
8 Likes
Related Questions
Define a class to perform binary search on a list of integers given below, to search for an element input by the user, if it is found display the element along with its position, otherwise display the message "Search element not found".
2, 5, 7, 10, 15, 20, 29, 30, 46, 50
Define a class to declare a character array of size ten. Accept the characters into the array and display the characters with highest and lowest ASCII (American Standard Code for Information Interchange) value.
EXAMPLE :
INPUT:
'R', 'z', 'q', 'A', 'N', 'p', 'm', 'U', 'Q', 'F'
OUTPUT :
Character with highest ASCII value = z
Character with lowest ASCII value = ADefine a class to accept a string, and print the characters with the uppercase and lowercase reversed, but all the other characters should remain the same as before.
EXAMPLE:
INPUT : WelCoMe2022 OUTPUT : wELcOmE2022Define a class to declare an array to accept and store ten words. Display only those words which begin with the letter 'A' or 'a' and also end with the letter 'A' or 'a'.
EXAMPLE :
Input : Hari, Anita, Akash, Amrita, Alina, Devi Rishab, John, Farha, AMITHA
Output: Anita
Amrita
Alina
AMITHA