Computer Applications
Define a class to declare a character array of size ten. Accept the characters into the array and display the characters with highest and lowest ASCII (American Standard Code for Information Interchange) value.
EXAMPLE :
INPUT:
'R', 'z', 'q', 'A', 'N', 'p', 'm', 'U', 'Q', 'F'
OUTPUT :
Character with highest ASCII value = z
Character with lowest ASCII value = A
Java
Java Arrays
ICSE 2022
25 Likes
Answer
import java.util.Scanner;
public class KboatASCIIVal
{
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
char ch[] = new char[10];
int len = ch.length;
System.out.println("Enter 10 characters:");
for (int i = 0; i < len; i++)
{
ch[i] = in.nextLine().charAt(0);
}
char h = ch[0];
char l = ch[0];
for (int i = 1; i < len; i++)
{
if (ch[i] > h)
{
h = ch[i];
}
if (ch[i] < l)
{
l = ch[i];
}
}
System.out.println("Character with highest ASCII value: " + h);
System.out.println("Character with lowest ASCII value: " + l);
}
}
Variable Description Table
Program Explanation
Output
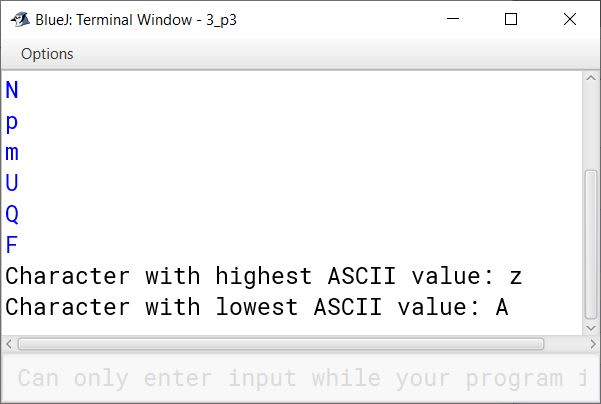
Answered By
10 Likes
Related Questions
What will be the output of the following code?
System.out.println("Lucknow".substring(0,4));
- Lucknow
- Luckn
- Luck
- luck
Define a class to perform binary search on a list of integers given below, to search for an element input by the user, if it is found display the element along with its position, otherwise display the message "Search element not found".
2, 5, 7, 10, 15, 20, 29, 30, 46, 50
Define a class to declare an array of size twenty of double datatype, accept the elements into the array and perform the following :
Calculate and print the product of all the elements.
Print the square of each element of the array.
Define a class to accept a string, and print the characters with the uppercase and lowercase reversed, but all the other characters should remain the same as before.
EXAMPLE:
INPUT : WelCoMe2022 OUTPUT : wELcOmE2022