Computer Applications
Define a class to overload the method display as follows:
void display( ): To print the following format using nested loop
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
void display(int n): To print the square root of each digit of the given number.
Example:
n = 4329
Output – 3.0
1.414213562
1.732050808
2.0
Java
Java Nested for Loops
ICSE Sp 2024
68 Likes
Answer
import java.util.Scanner;
public class KboatMethodOverload
{
public void display()
{
for(int i = 1; i <= 5; i++)
{
for(int j = 1; j <= i; j++)
{
System.out.print(j + " ");
}
System.out.println();
}
}
public void display(int n)
{
while( n != 0)
{
int d = n % 10;
System.out.println(Math.sqrt(d));
n = n / 10;
}
}
public static void main(String args[])
{
KboatMethodOverload obj = new KboatMethodOverload();
Scanner in = new Scanner(System.in);
System.out.println("Pattern: ");
obj.display();
System.out.print("Enter a number: ");
int num = in.nextInt();
obj.display(num);
}
}
Variable Description Table
Program Explanation
Output
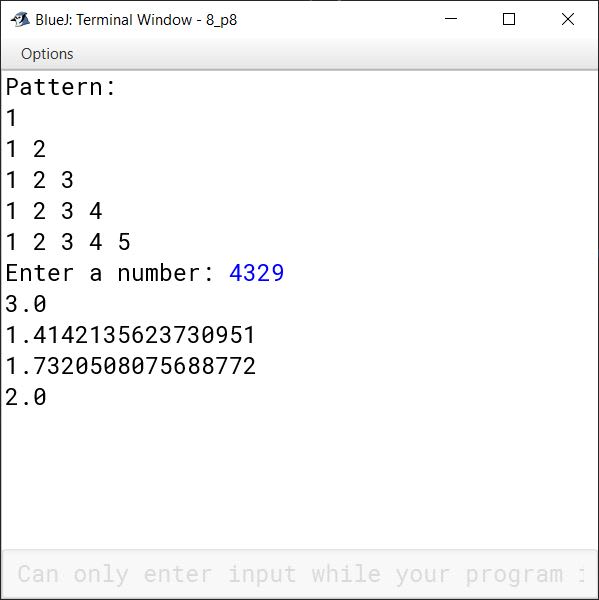
Answered By
37 Likes
Related Questions
Define a class to accept values in integer array of size 10. Sort them in an ascending order using selection sort technique. Display the sorted array.
Define a class to accept a string and convert it into uppercase. Count and display the number of vowels in it.
Input: robotics
Output: ROBOTICS
Number of vowels: 3Define a class to accept values into a 3 × 3 array and check if it is a special array. An array is a special array if the sum of the even elements = sum of the odd elements.
Example:
A[ ][ ]={{ 4 ,5, 6}, { 5 ,3, 2}, { 4, 2, 5}};
Sum of even elements = 4 + 6 + 2 + 4 + 2 = 18
Sum of odd elements = 5 + 5 + 3 + 5 = 18Define a class to accept a 3 digit number and check whether it is a duck number or not.
Note: A number is a duck number if it has zero in it.Example 1:
Input: 2083
Output: InvalidExample 2:
Input: 103
Output: Duck number