Computer Applications
Define a class to accept a string and convert it into uppercase. Count and display the number of vowels in it.
Input: robotics
Output: ROBOTICS
Number of vowels: 3
Java
Java String Handling
ICSE Sp 2024
74 Likes
Answer
import java.util.Scanner;
public class KboatCountVowels
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
System.out.print("Enter the string: ");
String str = in.nextLine();
str = str.toUpperCase();
str += " ";
int count = 0;
int len = str.length();
for (int i = 0; i < len - 1; i++)
{
char ch = str.charAt(i);
if(ch == 'A'
|| ch == 'E'
|| ch == 'I'
|| ch == 'O'
|| ch == 'U')
count++;
}
System.out.println("String : " + str);
System.out.println("Number of vowels : " + count);
}
}
Variable Description Table
Program Explanation
Output
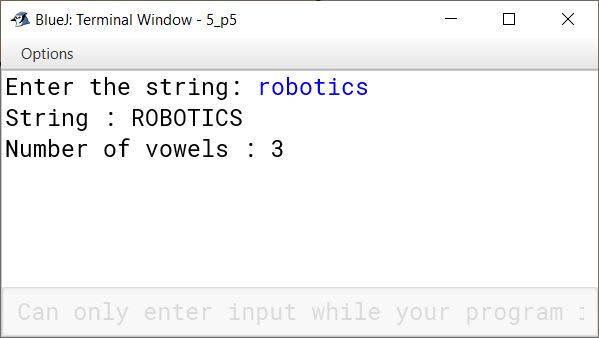
Answered By
30 Likes
Related Questions
Define a class called with the following specifications:
Class name: Eshop
Member variables:
String name: name of the item purchased
double price: Price of the item purchasedMember methods:
void accept(): Accept the name and the price of the item using the methods of Scanner class.
void calculate(): To calculate the net amount to be paid by a customer, based on the following criteria:Price Discount 1000 – 25000 5.0% 25001 – 57000 7.5 % 57001 – 100000 10.0% More than 100000 15.0 % void display(): To display the name of the item and the net amount to be paid.
Write the main method to create an object and call the above methods.
Define a class to accept values in integer array of size 10. Sort them in an ascending order using selection sort technique. Display the sorted array.
Define a class to accept values into a 3 × 3 array and check if it is a special array. An array is a special array if the sum of the even elements = sum of the odd elements.
Example:
A[ ][ ]={{ 4 ,5, 6}, { 5 ,3, 2}, { 4, 2, 5}};
Sum of even elements = 4 + 6 + 2 + 4 + 2 = 18
Sum of odd elements = 5 + 5 + 3 + 5 = 18Define a class to accept a 3 digit number and check whether it is a duck number or not.
Note: A number is a duck number if it has zero in it.Example 1:
Input: 2083
Output: InvalidExample 2:
Input: 103
Output: Duck number