Computer Applications
Define a class called with the following specifications:
Class name: Eshop
Member variables:
String name: name of the item purchased
double price: Price of the item purchased
Member methods:
void accept(): Accept the name and the price of the item using the methods of Scanner class.
void calculate(): To calculate the net amount to be paid by a customer, based on the following criteria:
Price | Discount |
---|---|
1000 – 25000 | 5.0% |
25001 – 57000 | 7.5 % |
57001 – 100000 | 10.0% |
More than 100000 | 15.0 % |
void display(): To display the name of the item and the net amount to be paid.
Write the main method to create an object and call the above methods.
Java
Java Classes
ICSE Sp 2024
157 Likes
Answer
import java.util.Scanner;
public class Eshop
{
private String name;
private double price;
private double disc;
private double amount;
public void accept() {
Scanner in = new Scanner(System.in);
System.out.print("Enter item name: ");
name = in.nextLine();
System.out.print("Enter price of item: ");
price = in.nextDouble();
}
public void calculate() {
double d = 0.0;
if (price < 1000)
d = 0.0;
else if (price <= 25000)
d = 5.0;
else if (price <= 57000)
d = 7.5;
else if (price <= 100000)
d = 10.0;
else
d = 15.0;
disc = price * d / 100.0;
amount = price - disc;
}
public void display() {
System.out.println("Item Name: " + name);
System.out.println("Net Amount: " + amount);
}
public static void main(String args[]) {
Eshop obj = new Eshop();
obj.accept();
obj.calculate();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
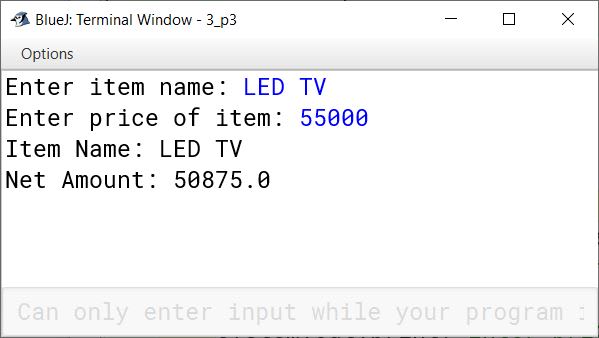
Answered By
70 Likes
Related Questions
When there is no explicit initialization, what are the default values set for variables in the following cases?
(a) Integer variable
(b) String variable
int P[ ] = {12, 14, 16, 18};
int Q[ ] = {20, 22, 24};Place all elements of P array and Q array in the array R one after the other.
(a) What will be the size of array R[ ] ?
(b) Write index position of first and last element?
Define a class to accept values in integer array of size 10. Sort them in an ascending order using selection sort technique. Display the sorted array.
Define a class to accept a string and convert it into uppercase. Count and display the number of vowels in it.
Input: robotics
Output: ROBOTICS
Number of vowels: 3