Computer Applications
Define a class to accept values in integer array of size 10. Sort them in an ascending order using selection sort technique. Display the sorted array.
Java
Java Arrays
ICSE Sp 2024
84 Likes
Answer
import java.util.Scanner;
public class KboatSelectionSort
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
int arr[] = new int[10];
System.out.println("Enter 10 integers: ");
for (int i = 0; i < 10; i++)
{
arr[i] = in.nextInt();
}
for (int i = 0; i < 9; i++)
{
int idx = i;
for (int j = i + 1; j < 10; j++)
{
if (arr[j] < arr[idx])
idx = j;
}
int t = arr[i];
arr[i] = arr[idx];
arr[idx] = t;
}
System.out.println("Sorted Array:");
for (int i = 0; i < 10; i++)
{
System.out.print(arr[i] + " ");
}
}
}
Variable Description Table
Program Explanation
Output
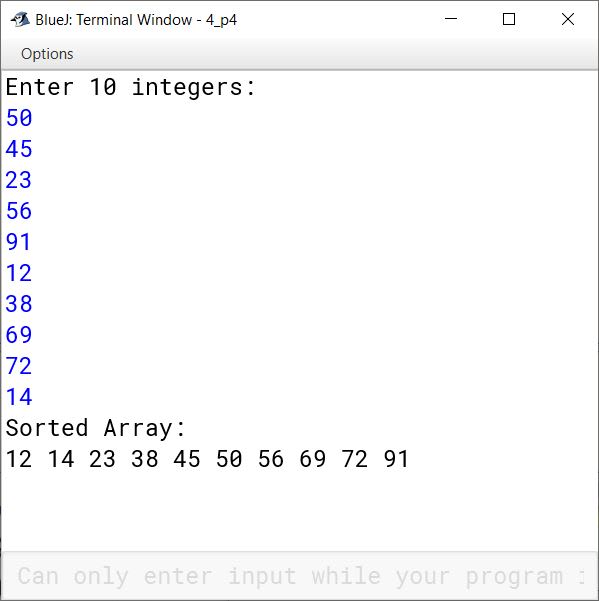
Answered By
36 Likes
Related Questions
int P[ ] = {12, 14, 16, 18};
int Q[ ] = {20, 22, 24};Place all elements of P array and Q array in the array R one after the other.
(a) What will be the size of array R[ ] ?
(b) Write index position of first and last element?
Define a class called with the following specifications:
Class name: Eshop
Member variables:
String name: name of the item purchased
double price: Price of the item purchasedMember methods:
void accept(): Accept the name and the price of the item using the methods of Scanner class.
void calculate(): To calculate the net amount to be paid by a customer, based on the following criteria:Price Discount 1000 – 25000 5.0% 25001 – 57000 7.5 % 57001 – 100000 10.0% More than 100000 15.0 % void display(): To display the name of the item and the net amount to be paid.
Write the main method to create an object and call the above methods.
Define a class to accept a string and convert it into uppercase. Count and display the number of vowels in it.
Input: robotics
Output: ROBOTICS
Number of vowels: 3Define a class to accept values into a 3 × 3 array and check if it is a special array. An array is a special array if the sum of the even elements = sum of the odd elements.
Example:
A[ ][ ]={{ 4 ,5, 6}, { 5 ,3, 2}, { 4, 2, 5}};
Sum of even elements = 4 + 6 + 2 + 4 + 2 = 18
Sum of odd elements = 5 + 5 + 3 + 5 = 18