Computer Applications
Define a class Employee having the following description:
Class name : Employee
Data Members | Purpose |
---|---|
int pan | To store personal account number |
String name | To store name |
double taxincome | To store annual taxable income |
double tax | To store tax that is calculated |
Member functions | Purpose |
---|---|
void input() | Store the pan number, name, taxable income |
void cal() | Calculate tax on taxable income |
void display() | Output details of an employee |
Calculate tax based on the given conditions and display the output as per the given format.
Total Annual Taxable Income | Tax Rate |
---|---|
Up to ₹2,50,000 | No tax |
From ₹2,50,001 to ₹5,00,000 | 10% of the income exceeding ₹2,50,000 |
From ₹5,00,001 to ₹10,00,000 | ₹30,000 + 20% of the income exceeding ₹5,00,000 |
Above ₹10,00,000 | ₹50,000 + 30% of the income exceeding ₹10,00,000 |
Output:
Pan Number Name Tax-Income Tax
.......... .... .......... ...
.......... .... .......... ...
Java
Java Classes
ICSE 2008
174 Likes
Answer
import java.util.Scanner;
public class Employee
{
private int pan;
private String name;
private double taxincome;
private double tax;
public void input() {
Scanner in = new Scanner(System.in);
System.out.print("Enter pan number: ");
pan = in.nextInt();
in.nextLine();
System.out.print("Enter Name: ");
name = in.nextLine();
System.out.print("Enter taxable income: ");
taxincome = in.nextDouble();
}
public void cal() {
if (taxincome <= 250000)
tax = 0;
else if (taxincome <= 500000)
tax = (taxincome - 250000) * 0.1;
else if (taxincome <= 1000000)
tax = 30000 + ((taxincome - 500000) * 0.2);
else
tax = 50000 + ((taxincome - 1000000) * 0.3);
}
public void display() {
System.out.println("Pan Number\tName\tTax-Income\tTax");
System.out.println(pan + "\t" + name + "\t"
+ taxincome + "\t" + tax);
}
public static void main(String args[]) {
Employee obj = new Employee();
obj.input();
obj.cal();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
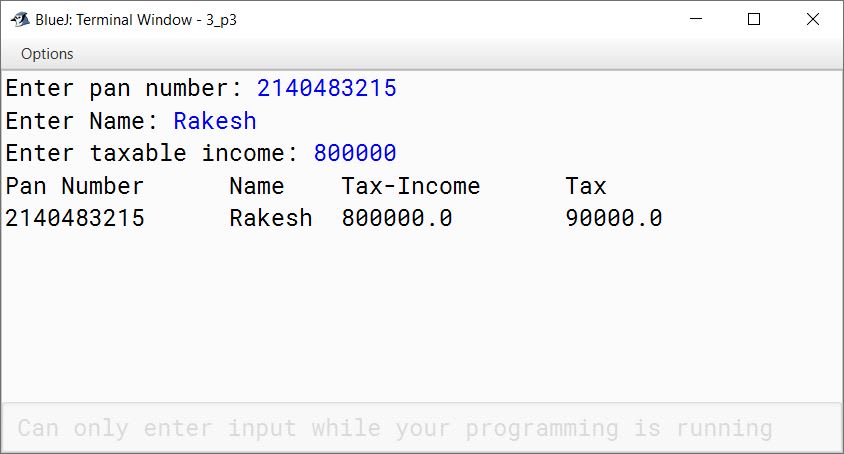
Answered By
75 Likes
Related Questions
Define a class Calculate to accept two numbers as instance variables. Use the following member methods for the given purposes:
Class name — Calculate
Data members — int a, int b
Member methods:
void inputdata() — to input both the values
void calculate() — to find sum and difference
void outputdata() — to print sum and difference of both the numbers
Use a main method to call the functions.Define a class Triplet with the following specifications:
Class name — Triplet
Data Members — int a, int b, int c
Member Methods:
void getdata() — to accept three numbers
void findprint() — to check and display whether the numbers are Pythagorean Triplets or not.Define a class Discount having the following description:
Class name : Discount
Data Members Purpose int cost to store the price of an article String name to store the customer's name double dc to store the discount double amt to store the amount to be paid Member methods Purpose void input() Stores the cost of the article and name of the customer void cal() Calculates the discount and amount to be paid void display() Displays the name of the customer, cost, discount and amount to be paid Write a program to compute the discount according to the given conditions and display the output as per the given format.
List Price Rate of discount Up to ₹5,000 No discount From ₹5,001 to ₹10,000 10% on the list price From ₹10,001 to ₹15,000 15% on the list price Above ₹15,000 20% on the list price Output:
Name of the customer Discount Amount to be paid .................... ........ ................. .................... ........ .................
Define a class Telephone having the following description:
Class name : Telephone
Data Members Purpose int prv, pre to store the previous and present meter readings int call to store the calls made (i.e. pre - prv) String name to store name of the consumer double amt to store the amount double total to store the total amount to be paid Member functions Purpose void input() Stores the previous reading, present reading and name of the consumer void cal() Calculates the amount and total amount to be paid void display() Displays the name of the consumer, calls made, amount and total amount to be paid Write a program to compute the monthly bill to be paid according to the given conditions and display the output as per the given format.
Calls made Rate Up to 100 calls No charge For the next 100 calls 90 paise per call For the next 200 calls 80 paise per call More than 400 calls 70 paise per call However, every consumer has to pay ₹180 per month as monthly rent for availing the service.
Output:
Name of the customer Calls made Amount to be paid .................... .......... ................. .................... .......... .................