Computer Applications
Define a class Calculate to accept two numbers as instance variables. Use the following member methods for the given purposes:
Class name — Calculate
Data members — int a, int b
Member methods:
void inputdata() — to input both the values
void calculate() — to find sum and difference
void outputdata() — to print sum and difference of both the numbers
Use a main method to call the functions.
Java
Java Classes
87 Likes
Answer
import java.util.Scanner;
public class Calculate
{
private int a;
private int b;
private int sum;
private int diff;
public void inputdata() {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
a = in.nextInt();
System.out.print("Enter second number: ");
b = in.nextInt();
}
public void calculate() {
sum = a + b;
diff = a - b;
}
public void outputdata() {
System.out.println("Sum = " + sum);
System.out.println("Difference = " + diff);
}
public static void main(String args[]) {
Calculate obj = new Calculate();
obj.inputdata();
obj.calculate();
obj.outputdata();
}
}
Variable Description Table
Program Explanation
Output
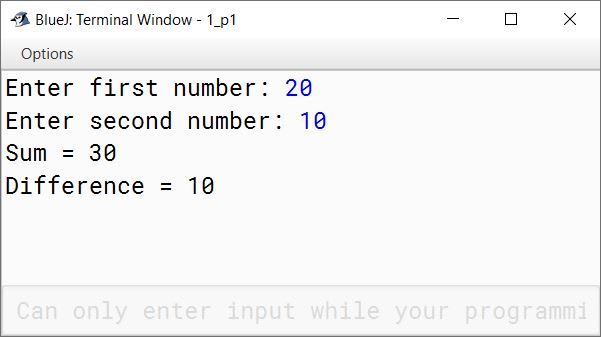
Answered By
40 Likes
Related Questions
Differentiate between private and protected visibility modifiers.
Differentiate between instance variable and class variable.
Define a class Triplet with the following specifications:
Class name — Triplet
Data Members — int a, int b, int c
Member Methods:
void getdata() — to accept three numbers
void findprint() — to check and display whether the numbers are Pythagorean Triplets or not.Define a class Employee having the following description:
Class name : Employee
Data Members Purpose int pan To store personal account number String name To store name double taxincome To store annual taxable income double tax To store tax that is calculated Member functions Purpose void input() Store the pan number, name, taxable income void cal() Calculate tax on taxable income void display() Output details of an employee Calculate tax based on the given conditions and display the output as per the given format.
Total Annual Taxable Income Tax Rate Up to ₹2,50,000 No tax From ₹2,50,001 to ₹5,00,000 10% of the income exceeding ₹2,50,000 From ₹5,00,001 to ₹10,00,000 ₹30,000 + 20% of the income exceeding ₹5,00,000 Above ₹10,00,000 ₹50,000 + 30% of the income exceeding ₹10,00,000 Output:
Pan Number Name Tax-Income Tax .......... .... .......... ... .......... .... .......... ...