Computer Applications
Define a class Discount having the following description:
Class name : Discount
Data Members | Purpose |
---|---|
int cost | to store the price of an article |
String name | to store the customer's name |
double dc | to store the discount |
double amt | to store the amount to be paid |
Member methods | Purpose |
---|---|
void input() | Stores the cost of the article and name of the customer |
void cal() | Calculates the discount and amount to be paid |
void display() | Displays the name of the customer, cost, discount and amount to be paid |
Write a program to compute the discount according to the given conditions and display the output as per the given format.
List Price | Rate of discount |
---|---|
Up to ₹5,000 | No discount |
From ₹5,001 to ₹10,000 | 10% on the list price |
From ₹10,001 to ₹15,000 | 15% on the list price |
Above ₹15,000 | 20% on the list price |
Output:
Name of the customer Discount Amount to be paid
.................... ........ .................
.................... ........ .................
Java
Java Classes
87 Likes
Answer
import java.util.Scanner;
public class Discount
{
private int cost;
private String name;
private double dc;
private double amt;
public void input() {
Scanner in = new Scanner(System.in);
System.out.print("Enter customer name: ");
name = in.nextLine();
System.out.print("Enter article cost: ");
cost = in.nextInt();
}
public void cal() {
if (cost <= 5000)
dc = 0;
else if (cost <= 10000)
dc = cost * 0.1;
else if (cost <= 15000)
dc = cost * 0.15;
else
dc = cost * 0.2;
amt = cost - dc;
}
public void display() {
System.out.println("Name of the customer\tDiscount\tAmount to be paid");
System.out.println(name + "\t" + dc + "\t" + amt);
}
public static void main(String args[]) {
Discount obj = new Discount();
obj.input();
obj.cal();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
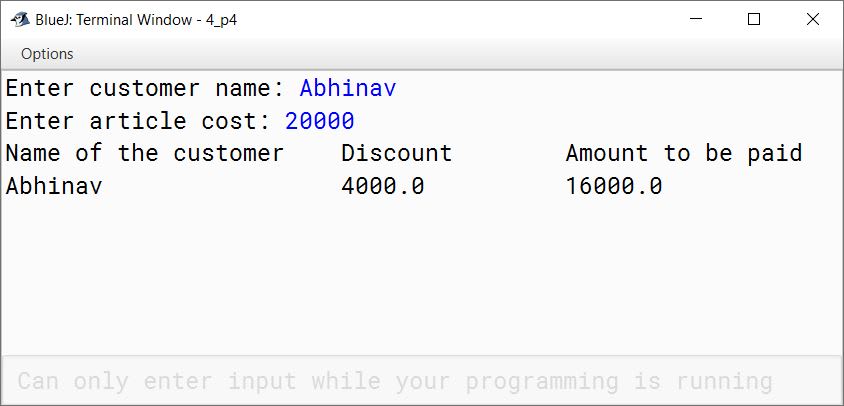
Answered By
44 Likes
Related Questions
Define a class Triplet with the following specifications:
Class name — Triplet
Data Members — int a, int b, int c
Member Methods:
void getdata() — to accept three numbers
void findprint() — to check and display whether the numbers are Pythagorean Triplets or not.Define a class Employee having the following description:
Class name : Employee
Data Members Purpose int pan To store personal account number String name To store name double taxincome To store annual taxable income double tax To store tax that is calculated Member functions Purpose void input() Store the pan number, name, taxable income void cal() Calculate tax on taxable income void display() Output details of an employee Calculate tax based on the given conditions and display the output as per the given format.
Total Annual Taxable Income Tax Rate Up to ₹2,50,000 No tax From ₹2,50,001 to ₹5,00,000 10% of the income exceeding ₹2,50,000 From ₹5,00,001 to ₹10,00,000 ₹30,000 + 20% of the income exceeding ₹5,00,000 Above ₹10,00,000 ₹50,000 + 30% of the income exceeding ₹10,00,000 Output:
Pan Number Name Tax-Income Tax .......... .... .......... ... .......... .... .......... ...
Define a class Telephone having the following description:
Class name : Telephone
Data Members Purpose int prv, pre to store the previous and present meter readings int call to store the calls made (i.e. pre - prv) String name to store name of the consumer double amt to store the amount double total to store the total amount to be paid Member functions Purpose void input() Stores the previous reading, present reading and name of the consumer void cal() Calculates the amount and total amount to be paid void display() Displays the name of the consumer, calls made, amount and total amount to be paid Write a program to compute the monthly bill to be paid according to the given conditions and display the output as per the given format.
Calls made Rate Up to 100 calls No charge For the next 100 calls 90 paise per call For the next 200 calls 80 paise per call More than 400 calls 70 paise per call However, every consumer has to pay ₹180 per month as monthly rent for availing the service.
Output:
Name of the customer Calls made Amount to be paid .................... .......... ................. .................... .......... .................
Define a class Interest having the following description:
Class name : Interest
Data Members Purpose int p to store principal (sum) int r to store rate int t to store time double interest to store the interest to be paid double amt to store the amount to be paid Member functions Purpose void input() Stores the principal, rate, time void cal() Calculates the interest and amount to be paid void display() Displays the principal, interest and amount to be paid Write a program to compute the interest according to the given conditions and display the output.
Time Rate of interest For 1 year 6.5% For 2 years 7.5% For 3 years 8.5% For 4 years or more 9.5% (Note: Time to be taken only in whole years)