Consider the below picture and choose the correct statement from the following:
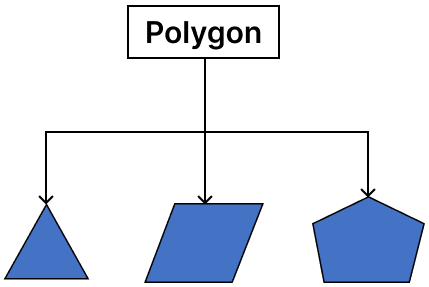
- Polygon is the object and the pictures are classes
- Both polygon and the pictures are classes
- Polygon is the class and the pictures are objects
- Both polygon and the pictures are objects
Answer
Polygon is the class and the pictures are objects
Reason — In object-oriented programming (OOP), a class is a blueprint or template that defines the properties and behaviours (methods) of objects. An object is an instance of a class that holds specific data defined by the class.
In this case:
- Polygon represents the class because it defines the general structure, properties, and methods common to all polygons (e.g., sides, vertices, perimeter calculation).
- The pictures are specific examples or instances of polygons, such as triangles, parallelogram, or pentagons, making them objects created from the Polygon class.
int x = 98; char ch = (char)x; what is the value in ch?
- b
- A
- B
- 97
Answer
b
Reason — In Java, when an int value is cast to char, it is converted based on the ASCII code. Since, 98 is the ASCII code of the letter b, hence value of ch is 'b'.
The output of the statement "CONCENTRATION".indexOf('T') is:
- 9
- 7
- 6
- (-1)
Answer
6
Reason — The method indexOf(char ch)
in Java returns the index of the first occurrence of the specified character ch
in the string. If the character is not found, it returns -1
.
Consider the string:
"CONCENTRATION"
Let’s count the characters by their index (Java uses zero-based indexing):
C | O | N | C | E | N | T | R | A | T | I | O | N |
---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 |
The first occurrence of the character 'T' is at index 6.
Thus, the output of the statement "CONCENTRATION".indexOf('T') is 6.
The access specifier that gives least accessibility is:
- package
- public
- protected
- private
Answer
private
Reason — In Java, access specifiers determine the visibility or accessibility of classes, methods, and variables. The three main access specifiers are:
public
: Accessible from anywhere in the program.protected
: Accessible within the same package and by subclasses even if they are in different packages.private
: Accessible only within the same class.
private
gives least accessibility as it provides the most restricted access.
The output of the statement "talent".compareTo("genius") is:
- 11
- –11
- 0
- 13
Answer
13
Reason — The method compareTo(String str)
in Java compares two strings lexicographically based on the ASCII values of characters. It returns:
0 if both strings are equal.
A positive value if the calling string comes after the argument string.
A negative value if the calling string comes before the argument string.
How It Works:
We compare "talent" and "genius" using their first differing characters:
- The ASCII value of
't'
= 116 - The ASCII value of
'g'
= 103
The difference is:
116 - 103 = 13
Since 't'
comes after 'g'
, the result is 13.
Which of the following is an escape sequence character in Java?
/n
\t
/t
//n
Answer
\t
Reason — In Java, an escape sequence is a combination of characters starting with a backslash (\
) followed by a specific character that performs a special operation.
Analysing the given options:
/n
– Incorrect, should be\n
(Backslash is required).\t
– Correct, it represents a tab./t
– Incorrect, should be\t
.//n
– Incorrect, this is not an escape sequence;//
is used for comments in Java.
if (a>b&&b>c) then largest number is:
- b
- c
- a
- wrong expression
Answer
a
Reason — The &&
(Logical AND) operator in Java is used to combine two boolean expressions. It returns true
only if both expressions are true
. If either of the expressions is false
, the entire condition becomes false
.
The condition if (a > b && b > c)
checks two expressions:
a > b
– Checks ifa
is greater thanb
.b > c
– Checks ifb
is greater thanc
.
Since both conditions must be true (due to the &&
operator), the logical sequence becomes:
a > b > c
In this sequence:a
is the largest number because it is greater than both b
and c
.
What is the output of Math.ceil(5.4)+Math.ceil(4.5)?
- 10.0
- 11.0
- 12.0
- 9.0
Answer
11.0
Reason — The method Math.ceil(double value)
in Java rounds up a decimal number to the next highest integer, regardless of the fractional part.
Breakdown of the Expression:
Math.ceil(5.4)
:
5.4 is rounded up to 6.0.Math.ceil(4.5)
:
4.5 is rounded up to 5.0.
Adding the Results:
6.0 + 5.0 = 11.0
What is the method to check whether a character is a letter or digit?
- isDigit(char)
- isLetterOrDigit()
- isLetterOrDigit(char)
- isLETTERorDIGIT(char)
Answer
isLetterOrDigit(char)
Reason — In Java, the method Character.isLetterOrDigit(char ch)
is used to check whether a given character ch
is either a letter (A-Z or a-z) or a digit (0-9).
Analysing Other Options:
isDigit(char)
– This method only checks if the character is a digit, not a letter.isLetterOrDigit()
– Incorrect because this method requires a character parameter.isLETTERorDIGIT(char)
– Java methods are case-sensitive, and method names follow camelCase. This method name does not exist.
The extension of a Java source code file is:
- exe
- obj
- jvm
- java
Answer
java
Reason — In Java, source code files are saved with the .java
extension. These files contain the Java program's source code, written in human-readable text format.
The number of bytes occupied by a character array of four rows and three columns are:
- 12
- 24
- 96
- 48
Answer
24
Reason — In Java, a character (char
) takes 2 bytes because Java uses Unicode encoding.
Given:
Character Array: 4 rows and 3 columns
Total Elements = 4 × 3 = 12 characters
Memory Calculation:
Each character = 2 bytes
Total Memory = 12 × 2 = 24 bytes
Which of the following data type cannot be used with switch case construct?
- int
- char
- String
- double
Answer
double
Reason — The switch
statement in Java supports specific data types for the case
labels. However, double
and float
data types cannot be used.
Data Types supported in switch
statement are:
int
char
String
Which of the following are entry controlled loops?
(a) for
(b) while
(c) do..while
(d) switch
- only a
- a and b
- a and c
- c and d
Answer
a and b
Reason — An entry-controlled loop checks the loop condition before executing the loop's body. If the condition is false at the start, the loop's body will not execute even once.
for and while loops check the condition before running the loop's body. If the condition is false, the loop won't run. Therefore, they are entry controlled loops.
The body of the do-while loop executes at least once before checking the condition. Hence, it is an exit controlled loop.
Method which reverses a given number is:
- Impure method
- Pure method
- Constructor
- Destructor
Answer
Pure method
Reason — A pure method in Java is a method that:
- Returns a value based only on its input arguments.
- Does not modify any external variables or system state.
- Does not change any class fields or input values.
In the context of reversing a number, a pure method accepts the number as input and returns its reversed form without altering any external state.
If the name of the class is "Yellow", what can be the possible name for its constructors?
- yellow
- YELLOW
- Yell
- Yellow
Answer
Yellow
Reason — In Java, a constructor must have the same name as the class. It initializes an object when it is created. Since the class name is Yellow
, the constructor's name must be exactly Yellow
, matching the class name including capitalization.
Invoking a method by passing the objects of a class is termed as:
- Call by reference
- Call by value
- Call by method
- Call by constructor
Answer
Call by reference
Reason — When an object is passed to a method:
- The method receives a reference (memory address) to the original object.
- Any changes made inside the method affect the original object.
The correct statement to create an object named mango of class fruit:
- Fruit Mango= new fruit();
- fruit mango = new fruit();
- Mango fruit=new Mango();
- fruit mango= new mango();
Answer
fruit mango = new fruit();
Reason — In Java, creating an object follows the syntax:
ClassName objectName = new ClassName();
Where:
ClassName
: The name of the class.objectName
: The name of the object.new
: Allocates memory for the object.ClassName()
: Calls the class's constructor.
Thus, fruit mango = new fruit();
correctly creates mango named object of fruit class.
Assertion (A): Static method can access static and instance variables.
Reason (R): Static variable can be accessed only by static method.
- Assertion and Reason both are correct.
- Assertion is true and Reason is false.
- Assertion is false and Reason is true.
- Assertion and Reason both are false.
Answer
Assertion and Reason both are false.
Reason — A static method can only access static variables and static methods directly because it belongs to the class, not any specific object. Hence, Assertion is false.
Both instance and static methods can access static variables. Hence, Reason is false.
What is the output of the Java code given below?
String color[] = {"Blue", "Red", "Violet"};
System.out.println(color[2].length());
- 6
- 5
- 3
- 2
Answer
6
Reason — The given Java code declares and initializes a String array color
with three elements. The array elements are:
color[0] = "Blue"
color[1] = "Red"
color[2] = "Violet"
The method length()
returns the number of characters in the string "Violet"
.
Which of the following mathematical methods returns only an integer?
- Math.ceil(n)
- Math.sqrt(n)
- Math.floor(n)
- Math.round(n)
Answer
Math.round(n)
Reason — In Java, Math.round(n)
is the only method in the list that returns an integer when called with a floating-point number (float
or double
).
Write Java expression for:
Answer
The Java expression for the given mathematical expression can be written as:
double result = Math.abs(a + b) / Math.sqrt(Math.pow(a, 2) + Math.pow(b, 2));
Evaluate the expression when x is 4:
x += x++ * ++x % 2;
Answer
The given expression is evaluated as follows:
x += x++ * ++x % 2
x = x + (x++ * ++x % 2) (x = 4)
x = 4 + (4 * ++x % 2) (x = 5)
x = 4 + (4 * 6 % 2) (x = 6)
x = 4 + (24 % 2) (x = 6)
x = 4 + 0 (x = 6)
x = 4
Rewrite the following do while program segment using for:
x = 10; y = 20;
do
{
x++;
y++;
} while (x<=20);
System.out.println(x * y );
Answer
Below is the for
loop version of the provided do-while
segment:
public class Main {
public static void main(String[] args) {
int x = 10, y = 20;
for (; x <= 20; x++, y++) {
// Body is empty because the increments are in the for loop header
}
System.out.println(x * y);
}
}
Give the output of the following program segment. How many times is the loop executed?
for(x=10; x>20;x++)
System.out.println(x);
System.out.println(x*2);
Answer
20
The loop executes 0 times because the loop condition x>20
is false as value of x is 10.
String s1 = "45.50"; String s2 = "54.50";
double d1=Double.parseDouble(s1);
double d2=Double.parseDouble(s2);
int x= (int)(d1+d2);
What is value of x?
Answer
100
Reason — The code converts the strings "45.50"
and "54.50"
into doubles using Double.parseDouble()
, resulting in d1 = 45.5
and d2 = 54.5
. Their sum is 100.0
.
Casting 100.0
to an integer using (int)
removes the decimal part, leaving x = 100
.
Consider the following two-dimensional array and answer the questions given below:
int x[ ][ ] = {{4,3,2}, {7,8,2}, {8, 3,10}, {1, 2, 9}};
(a) What is the order of the array?
(b) What is the value of x[0][0]+x[2][2]?
Answer
(a) The order of the array is 4 x 3 as it has 4 rows and 3 columns.
(b) The value is evaluated as follows:
x[0][0] = 4
x[2][2] = 10
x[0][0]+x[2][2] = 4 + 10 = 14
Differentiate between boxing and unboxing.
Answer
Differences between boxing and unboxing are:
Boxing | Unboxing |
---|---|
It is the process of converting a primitive type to its corresponding wrapper class object. | It is the process of converting a wrapper class object back to its corresponding primitive type. |
It occurs when assigning a primitive value to a wrapper object. | It occurs when assigning a wrapper object to a primitive variable. |
Example: int num = 10; Integer obj = num; | Example: Integer obj = 20; int num = obj; |
The following code to compare two strings is compiled, the following syntax error was displayed – incompatible types – int cannot be converted to boolean.
Identify the statement which has the error and write the correct statement. Give the output of the program segment.
void calculate()
{
String a = "KING", b = "KINGDOM";
boolean x = a.compareTo(b);
System.out.println(x);
}
Answer
boolean x = a.compareTo(b);
has the error.
Corrected statement is:
boolean x = (a.compareTo(b) == 0);
false
Explanation
The error occurs in this line:
boolean x = a.compareTo(b);
- The
compareTo()
method of theString
class returns anint
, not aboolean
. - The method compares two strings lexicographically and returns:
- 0 if the strings are equal.
- A positive value if the calling string is lexicographically greater.
- A negative value if the calling string is lexicographically smaller.
Since an int
cannot be assigned to a boolean
variable, this causes a "incompatible types" error.
To fix the error, we can use a comparison to convert the int
result into a boolean
:
boolean x = (a.compareTo(b) == 0); // True if strings are equal
This assigns true
or false
based on whether the two strings are equal.
Corrected Program:
void calculate() {
String a = "KING", b = "KINGDOM";
boolean x = (a.compareTo(b) == 0); // Check if strings are equal
System.out.println(x);
}
Output of the Program:
"KING".compareTo("KINGDOM")
compares the strings lexicographically."KING"
is lexicographically smaller than"KINGDOM"
.- The result of
compareTo()
will be -3 (difference between the lengths of the two strings ⇒ 4 - 7 = -3).
Since a.compareTo(b) == 0
is false, the output will be:
false
Consider the given program and answer the questions given below:
class temp
{
int a;
temp()
{
a=10;
}
temp(int z)
{
a=z;
}
void print()
{
System.out.println(a);
}
void main()
{
temp t = new temp();
temp x = new temp(30);
t.print();
x.print();
}
}
(a) What concept of OOPs is depicted in the above program with two constructors?
(b) What is the output of the method main()?
Answer
(a) The concept of Constructor Overloading (Polymorphism) is depicted in the program.
(b) Output of the main() method:
10
30
Explanation:
Output of the program is explained below:
temp t = new temp();
Calls the default constructortemp()
→a = 10
temp x = new temp(30);
Calls the parameterized constructortemp(int z)
→a = 30
t.print();
Prints value ofa
fort
→10
x.print();
Prints value ofa
forx
→30
Primitive data types are built in data types which are a part of the wrapper classes. These wrapper classes are encapsulated in the java.lang package. Non primitive datatypes like Scanner class are a part of the utility package for which an object needs to be created.
(a) To which package the Character and Boolean classes belong?
(b) Write the statement to access the Scanner class in the program.
Answer
(a) java.lang
(b) import java.util.Scanner
DTDC a courier company charges for the courier based on the weight of the parcel. Define a class with the following specifications:
Class name: courier
Member variables:
name – name of the customer
weight – weight of the parcel in kilograms
address – address of the recipient
bill – amount to be paid
type – 'D'- domestic, 'I'- international
Member methods:
void accept ( ) — to accept the details using the methods of the Scanner class only.
void calculate ( ) — to calculate the bill as per the following criteria:
Weight in Kgs | Rate per Kg |
---|---|
First 5 Kgs | Rs.800 |
Next 5 Kgs | Rs.700 |
Above 10 Kgs | Rs.500 |
An additional amount of Rs.1500 is charged if the type of the courier is I (International)
void print ( ) — To print the details
void main ( ) — to create an object of the class and invoke the methods
import java.util.Scanner;
public class courier
{
private String name;
private double weight;
private String address;
private double bill;
private char type;
public void accept() {
Scanner in = new Scanner(System.in);
System.out.print("Enter customer's name: ");
name = in.nextLine();
System.out.print("Enter recipient's address: ");
address = in.nextLine();
System.out.print("Enter parcel weight (in Kgs): ");
weight = in.nextDouble();
System.out.println("Enter courier type");
System.out.print("D (Domestic), I (International): ");
type = in.next().charAt(0);
}
public void calculate() {
if (weight <= 5) {
bill = weight * 800;
} else if (weight <= 10) {
bill = (5 * 800) + ((weight - 5) * 700);
} else {
bill = (5 * 800) + (5 * 700) + ((weight - 10) * 500);
}
if (type == 'I') {
bill += 1500;
}
}
public void print() {
System.out.println("Customer's Name: " + name);
System.out.println("Parcel Weight: " + weight + " Kgs");
System.out.println("Recipient's Address: " + address);
System.out.println("Type of Courier: " + type);
System.out.println("Bill Amount: Rs." + bill);
}
public static void main(String[] args) {
courier obj = new courier();
obj.accept();
obj.calculate();
obj.print();
}
}
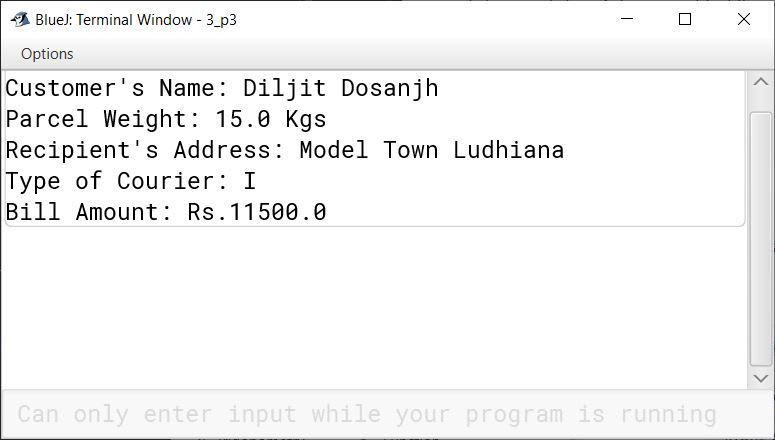
Define a class to overload the method perform as follows:
double perform (double r, double h) — to calculate and return the value of curved surface area of cone
void perform (int r, int c) — Use NESTED FOR LOOP to generate the following format
r = 4, c = 5
output
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
1 2 3 4 5
void perform (int m, int n, char ch) — to print the quotient of the division of m and n if ch is Q else print the remainder of the division of m and n if ch is R
Answer
import java.util.Scanner;
public class KboatOverloadPerform
{
double perform(double r, double h) {
double l = Math.sqrt((r * r) + (h * h));
double csa = Math.PI * r * l;
return csa;
}
void perform(int r, int c) {
for (int i = 1; i <= r; i++) {
for (int j = 1; j <= c; j++) {
System.out.print(j + " ");
}
System.out.println();
}
}
void perform(int m, int n, char ch) {
if (ch == 'Q') {
int q = m / n;
System.out.println("Quotient: " + q);
} else if (ch == 'R') {
int r = m % n;
System.out.println("Remainder: " + r);
} else {
System.out.println("Invalid Character!");
}
}
public static void main(String[] args) {
KboatOverloadPerform mo = new KboatOverloadPerform();
// Calculating CSA of a cone
double csa = mo.perform(3.0, 4.0);
System.out.println("Curved Surface Area of Cone: " + csa);
// Generating pattern
mo.perform(4, 5);
// Printing quotient or remainder
mo.perform(20, 6, 'Q');
mo.perform(20, 6, 'R');
}
}
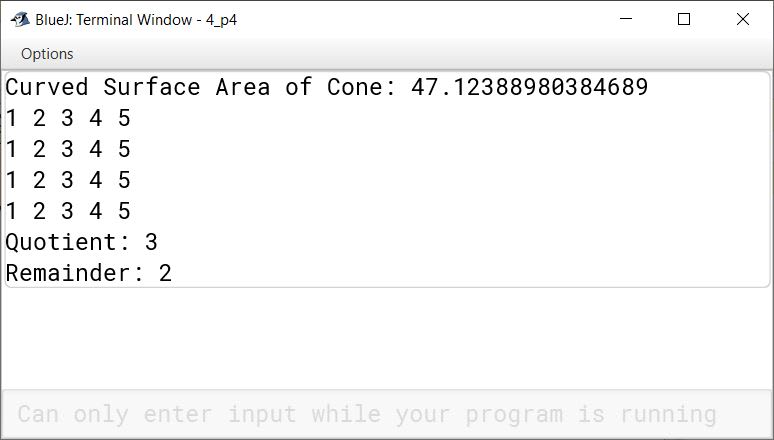
Define a class to accept a number from user and check if it is an EvenPal number or not.
(The number is said to be EvenPal number when number is palindrome number (a number is palindrome if it is equal to its reverse) and sum of its digits is an even number.)
Example: 121 – is a palindrome number
Sum of the digits – 1+2+1 = 4 which is an even number
Answer
import java.util.Scanner;
public class KboatEvenPal
{
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = in.nextInt();
int org = num;
int rev = 0;
int sum = 0;
while (num > 0) {
int d = num % 10;
rev = rev * 10 + d;
sum += d;
num /= 10;
}
if (org == rev && sum % 2 == 0) {
System.out.println(org + " is an EvenPal number.");
} else {
System.out.println(org + " is not an EvenPal number.");
}
}
}
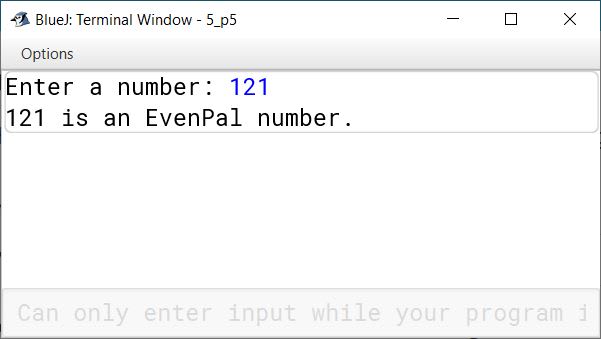
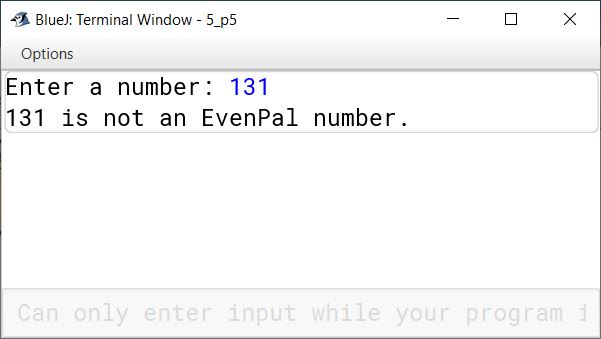
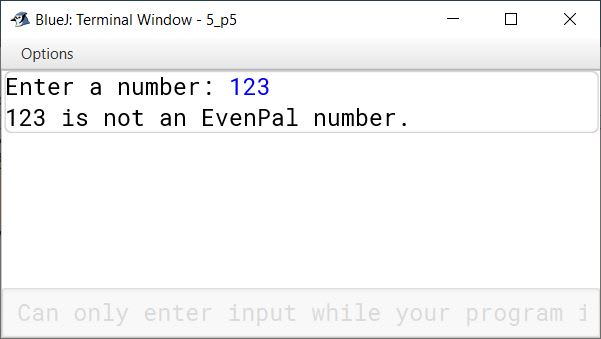
Define a class to accept values into an integer array of order 4 x 4 and check whether it is a DIAGONAL array or not. An array is DIAGONAL if the sum of the left diagonal elements equals the sum of the right diagonal elements. Print the appropriate message.
Example:
3 4 2 5
2 5 2 3
5 3 2 7
1 3 7 1
Sum of the left diagonal element = 3 + 5 + 2 + 1 = 11
Sum of the right diagonal element = 5 + 2 + 3 + 1 = 11
Answer
import java.util.Scanner;
public class KboatDiagonalDDA
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int[][] arr = new int[4][4];
System.out.println("Enter elements for 4x4 DDA:");
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
arr[i][j] = in.nextInt();
}
}
int lSum = 0;
int rSum = 0;
for (int i = 0; i < 4; i++) {
lSum += arr[i][i];
rSum += arr[i][3 - i];
}
if (lSum == rSum) {
System.out.println("The array is a DIAGONAL array.");
} else {
System.out.println("The array is NOT a DIAGONAL array.");
}
}
}
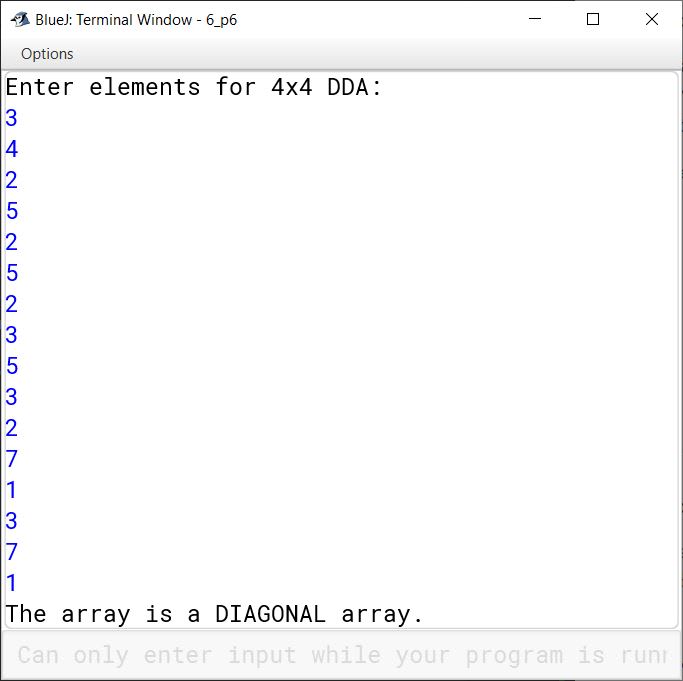
Define a class pin code and store the given pin codes in a single dimensional array. Sort these pin codes in ascending order using the Selection Sort technique only. Display the sorted array.
110061, 110001, 110029, 110023, 110055, 110006, 110019, 110033
Answer
public class Pincode
{
public static void main(String args[]) {
int[] arr = {110061, 110001,
110029, 110023,
110055, 110006,
110019, 110033};
for (int i = 0; i < 7; i++)
{
int idx = i;
for (int j = i + 1; j < 8; j++)
{
if (arr[j] < arr[idx])
idx = j;
}
int t = arr[i];
arr[i] = arr[idx];
arr[idx] = t;
}
System.out.println("Sorted Array:");
for (int i = 0; i < 8; i++)
{
System.out.print(arr[i] + " ");
}
}
}
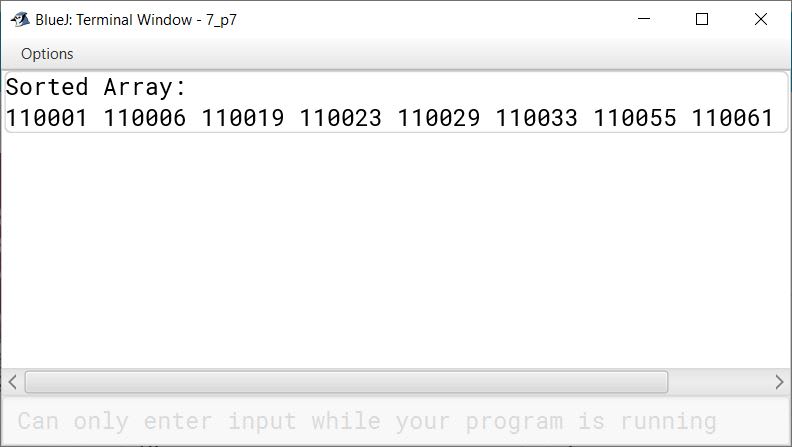
Define a class to accept the gmail id and check for its validity.
A gmail id is valid only if it has:
→ @
→ .(dot)
→ gmail
→ com
Example: icse2024@gmail.com
is a valid gmail id
Answer
import java.util.Scanner;
public class KboatValidateGmail
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
System.out.print("Enter a Gmail ID: ");
String gmailId = in.nextLine();
int len = gmailId.length();
if (gmailId.endsWith("@gmail.com")
&& gmailId.indexOf('@') == len - 10)
{
System.out.println(gmailId + " is a valid Gmail ID.");
}
else
{
System.out.println(gmailId + " is NOT a valid Gmail ID.");
}
}
}
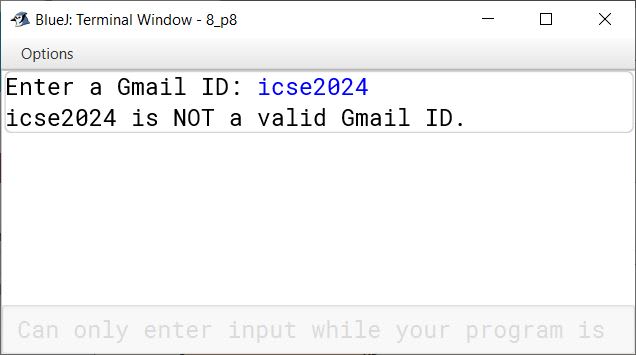
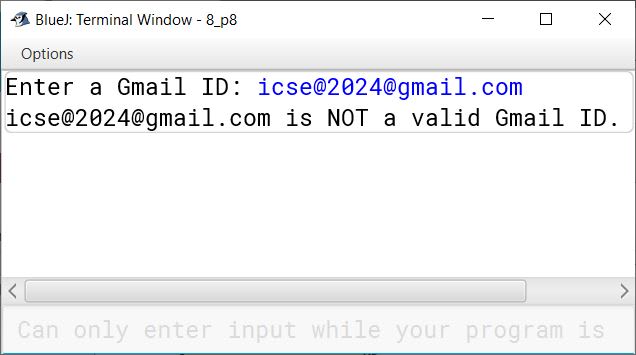
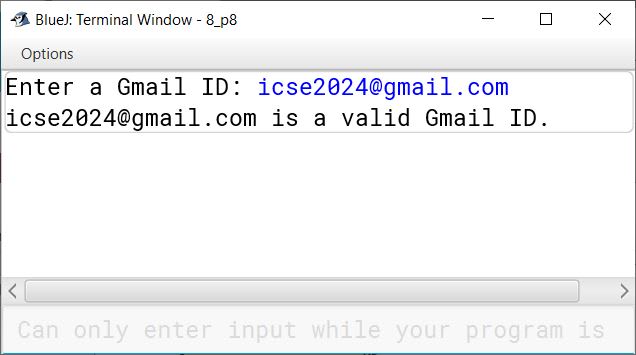