Computer Applications
Write three different programs using for, while, and do-while loops to find the product of series 3, 9, 12,… 30.
Java Iterative Stmts
4 Likes
Answer
for loop
public class KBoatSeries
{
public static void main(String args[]) {
int pro = 1;
for(int i = 3, t = 1; i <= 30; t++) {
pro *= i;
if(t % 2 == 0)
i += 3;
else
i += 6;
}
System.out.println("Product = " + pro);
}
}
Output
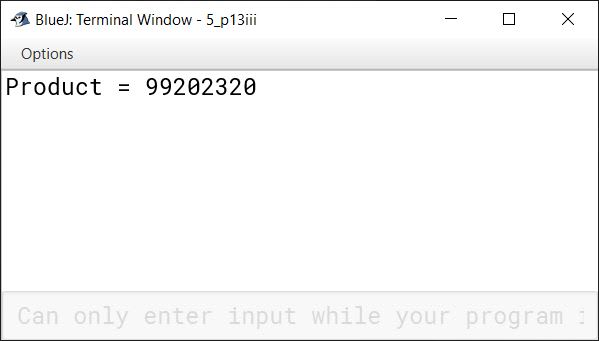
while loop
public class KBoatSeries
{
public static void main(String args[]) {
long pro = 1;
int num = 3, t = 1;
while(num <= 30) {
pro *= num;
if(t % 2 == 0)
num += 3;
else
num += 6;
t++;
}
System.out.println("Product = " + pro);
}
}
Output
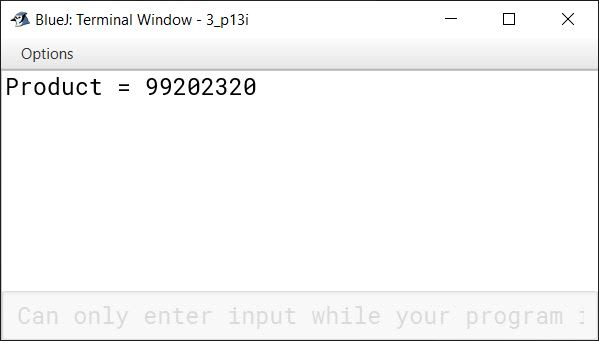
do-while loop
public class KBoatSeries
{
public static void main(String args[]) {
long pro = 1;
int num = 3, t = 1;
do {
pro *= num;
if(t % 2 == 0)
num += 3;
else
num += 6;
t++;
} while(num <= 30);
System.out.println("Product = " + pro);
}
}
Output
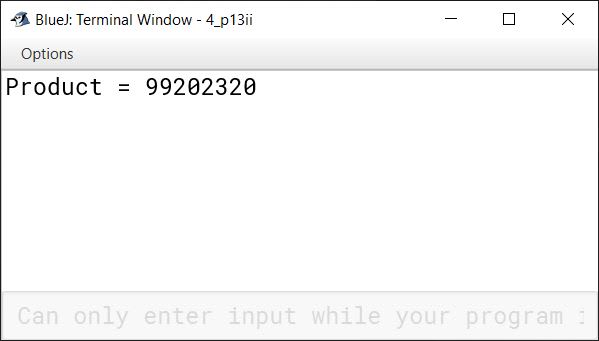
Answered By
3 Likes
Related Questions
Write a program to input n number of integers and find out:
i. Number of positive integers
ii. Number of negative integers
iii. Sum of positive numbers
iv. Product of negative numbers
v. Average of positive numbers
Write a program using do-while loop to compute the sum of the first 500 positive odd integers.
Write a program to convert kilograms to pounds in the following tabular format (1 kilogram is 2.2 pounds):
Kilograms Pounds 1 2.2 2 4.4 20 44.0 Write a program that displays all the numbers from 150 to 250 that are divisible by 5 or 6, but not both.