Computer Applications
Write a program to input n number of integers and find out:
i. Number of positive integers
ii. Number of negative integers
iii. Sum of positive numbers
iv. Product of negative numbers
v. Average of positive numbers
Java
Java Iterative Stmts
2 Likes
Answer
import java.util.Scanner;
public class KboatIntegers
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int pSum = 0, pCount = 0, nPro = 1, nCount = 0;
System.out.println("Enter n:");
int n = in.nextInt();
System.out.println("Enter " + n + " integers:");
for (int i = 1; i <= n; i++) {
int num = in.nextInt();
if (num >= 0) {
pSum += num;
pCount++;
}
else {
nPro *= num;
nCount++;
}
}
double pAvg = pSum/(double)pCount;
System.out.println("Positive Integers = " + pCount);
System.out.println("Negative Integers = " + nCount);
System.out.println("Sum of Positive = " + pSum);
System.out.println("Product of Negative = " + nPro);
System.out.println("Average of Positive = " + pAvg);
}
}
Output
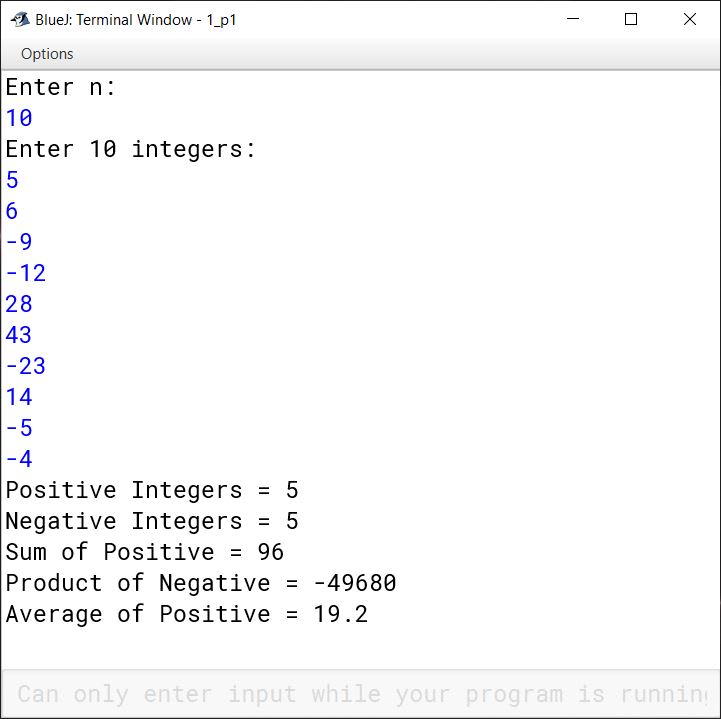
Answered By
1 Like
Related Questions
Write a program using do-while loop to compute the sum of the first 500 positive odd integers.
Write three different programs using for, while, and do-while loops to find the product of series 3, 9, 12,… 30.
What is the output produced by the following code?
int num = 20; while (num > 0) { num = num - 2; if (num == 4) break ; System.out.println(num); } System.out.println("Finished");
What is the output produced by the following code?
int num = 10; while (num > 0) { num = num - 2; if (num == 2) continue; System.out.println(num); } System.out.println("Finished");