Computer Applications
Write a program, which will get text string and count all occurrences of a particular word.
Java
Java String Handling
1 Like
Answer
import java.util.Scanner;
public class KboatWordFrequency
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
System.out.println("Enter a word:");
String ipWord = in.nextLine();
str += " ";
String word = "";
int count = 0;
int len = str.length();
for (int i = 0; i < len; i++) {
if (str.charAt(i) == ' ') {
if (word.equalsIgnoreCase(ipWord))
count++ ;
word = "";
}
else {
word += str.charAt(i);
}
}
if (count > 0) {
System.out.println(ipWord + " is present " + count + " times.");
}
else {
System.out.println(ipWord + " is not present in sentence.");
}
}
}
Variable Description Table
Program Explanation
Output
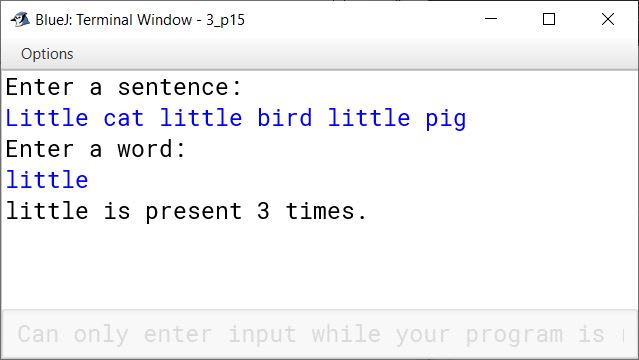
Answered By
3 Likes
Related Questions
Write a program to extract a portion of a character string and print the extracted string. Assume that m characters are extracted, starting with the nth character.
Write a program to accept a string. Convert the string into upper case letters. Count and output the number of double letter sequences that exist in the string.
Sample Input: "SHE WAS FEEDING THE LITTLE RABBIT WITH AN APPLE"
Sample Output: 4Design a class to overload a function check( ) as follows:
void check (String str , char ch ) — to find and print the frequency of a character in a string.
Example:
Input:
str = "success"
ch = 's'
Output:
number of s present is = 3void check(String s1) — to display only vowels from string s1, after converting it to lower case.
Example:
Input:
s1 ="computer"
Output : o u e
Write a program to do the following :
(a) To output the question "Who is the inventor of Java" ?
(b) To accept an answer.
(c) To print out "Good" and then stop, if the answer is correct.
(d) To output the message "try again", if the answer is wrong.
(e) To display the correct answer when the answer is wrong even at the third attempt and stop.