Computer Applications
Write a program to extract a portion of a character string and print the extracted string. Assume that m characters are extracted, starting with the nth character.
Java
Java String Handling
15 Likes
Answer
import java.util.Scanner;
public class KboatExtractSubstring
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
System.out.print("Enter string : ");
String s = in.nextLine();
int len = s.length();
System.out.print("Enter start index : ");
int n = in.nextInt();
if(n < 0 || n >= len)
{
System.out.println("Invalid index");
System.exit(1);
}
System.out.print("Enter no. of characters to extract : ");
int m = in.nextInt();
int substrlen = n + m;
if( m <= 0 || substrlen > len)
{
System.out.println("Invalid no. of characters");
System.exit(1);
}
String substr = s.substring(n, substrlen);
System.out.println("Substring = " + substr);
}
}
Variable Description Table
Program Explanation
Output
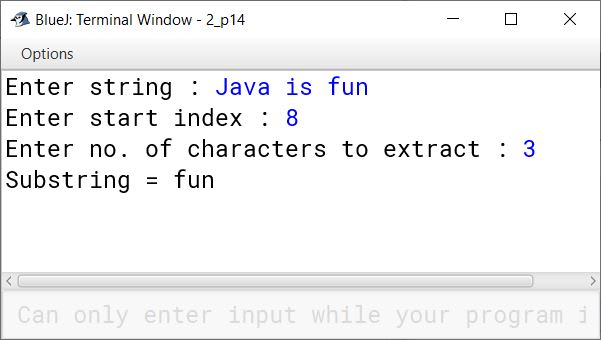
Answered By
5 Likes
Related Questions
Give the output of the following program :
class MainString { public static void main(String[ ] args) { StringBuffer s = new StringBuffer("String"); if((s.length( ) > 5) && (s.append("Buffer").equals("X"))) ; // empty statement System.out.println(s); } }
Write a program to do the following :
(a) To output the question "Who is the inventor of Java" ?
(b) To accept an answer.
(c) To print out "Good" and then stop, if the answer is correct.
(d) To output the message "try again", if the answer is wrong.
(e) To display the correct answer when the answer is wrong even at the third attempt and stop.
Write a program, which will get text string and count all occurrences of a particular word.
Write a program to accept a string. Convert the string into upper case letters. Count and output the number of double letter sequences that exist in the string.
Sample Input: "SHE WAS FEEDING THE LITTLE RABBIT WITH AN APPLE"
Sample Output: 4