Computer Applications
Write a program using a class with the following specifications:
Class name: Caseconvert
Data Members | Purpose |
---|---|
String str | To store the string |
Member Methods | Purpose |
---|---|
void getstr() | to accept a string |
void convert() | to obtain a string after converting each upper case letter into lower case and vice versa |
void display() | to print the converted string |
Java
Java Classes
Answer
import java.util.Scanner;
public class Caseconvert
{
private String str;
private String convStr;
public void getstr() {
Scanner in = new Scanner(System.in);
System.out.print("Enter the string: ");
str = in.nextLine();
}
public void convert() {
char arr[] = new char[str.length()];
for (int i = 0; i < str.length(); i++) {
if (Character.isUpperCase(str.charAt(i)))
arr[i] = Character.toLowerCase(str.charAt(i));
else if (Character.isLowerCase(str.charAt(i)))
arr[i] = Character.toUpperCase(str.charAt(i));
else
arr[i] = str.charAt(i);
}
convStr = new String(arr);
}
public void display() {
System.out.println("Converted String:");
System.out.println(convStr);
}
public static void main(String args[]) {
Caseconvert obj = new Caseconvert();
obj.getstr();
obj.convert();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
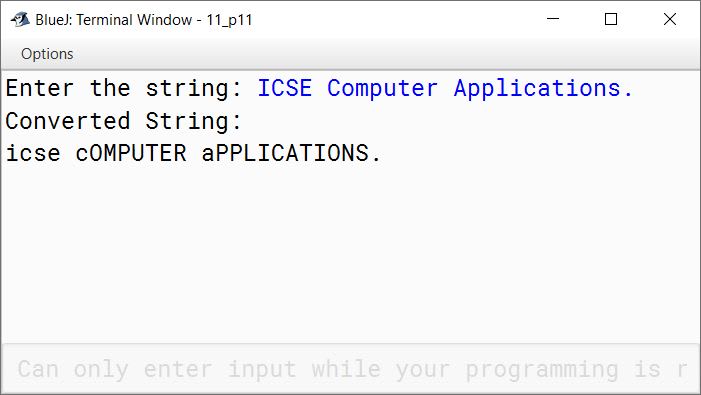
Answered By
Related Questions
Hero Honda has increased the cost of its vehicles as per the type of the engine using the following criteria:
Type of Engine Rate of increment 2 stroke 10% of the cost 4 stroke 12% of the cost Write a program by using a class to find the new cost as per the given specifications:
Class name: Honda
Data Members Purpose int type To accept type of engine 2 stroke or 4 stroke int cost To accept previous cost Member Methods Purpose void gettype() To accept the type of engine and previous cost void find() To find the new cost as per the criteria given above void printcost() To print the type and new cost of the vehicle Define a class called 'Mobike' with the following specifications:
Data Members Purpose int bno To store the bike number int phno To store the phone number of the customer String name To store the name of the customer int days To store the number of days the bike is taken on rent int charge To calculate and store the rental charge Member Methods Purpose void input() To input and store the details of the customer void compute() To compute the rental charge void display() To display the details in the given format The rent for a mobike is charged on the following basis:
Days Charge For first five days ₹500 per day For next five days ₹400 per day Rest of the days ₹200 per day Output:
Bike No. Phone No. Name No. of days Charge xxxxxxx xxxxxxxx xxxx xxx xxxxxx
Write a program by using a class with the following specifications:
Class name: Vowel
Data Members Purpose String s To store the string int c To count vowels Member Methods Purpose void getstr() to accept a string void getvowel() to count the number of vowels void display() to print the number of vowels A bookseller maintains record of books belonging to the various publishers. He uses a class with the specifications given below:
Class name — Stock
Data Members:
- String title — Contains title of the book
- String author — Contains author name
- String pub — Contains publisher's name
- int noc — Number of copies
Member Methods:
- void getdata() — To accept title, author, publisher's name and the number of copies.
- void purchase(int t, String a, String p, int n) — To check the existence of the book in the stock by comparing total, author's and publisher's name. Also check whether noc >n or not. If yes, maintain the balance as noc-n, otherwise display book is not available or stock is under flowing.
Write a program to perform the task given above.