Computer Applications
Write a program to search for an integer value input by the user in the list given below using linear search technique. If found display "Search Successful" and print the index of the element in the array, otherwise display "Search Unsuccessful".
{75, 86, 90, 45, 31, 50, 36, 60, 12, 47}
Java
Java Arrays
65 Likes
Answer
import java.util.Scanner;
public class KboatLinearSearch
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = {75, 86, 90, 45, 31, 50, 36, 60, 12, 47};
int l = arr.length;
int i = 0;
System.out.print("Enter the number to search: ");
int n = in.nextInt();
for (i = 0; i < l; i++) {
if (arr[i] == n) {
break;
}
}
if (i == l) {
System.out.println("Search Unsuccessful");
}
else {
System.out.println("Search Successful");
System.out.println(n + " present at index " + i);
}
}
}
Output
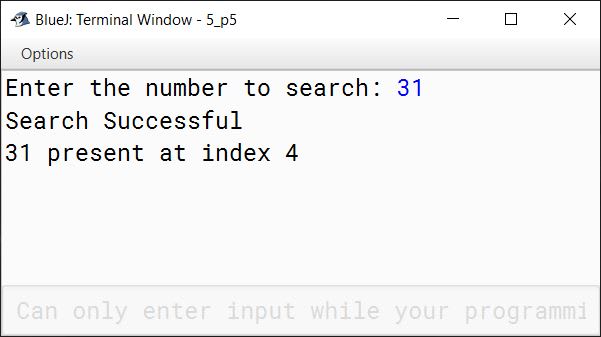
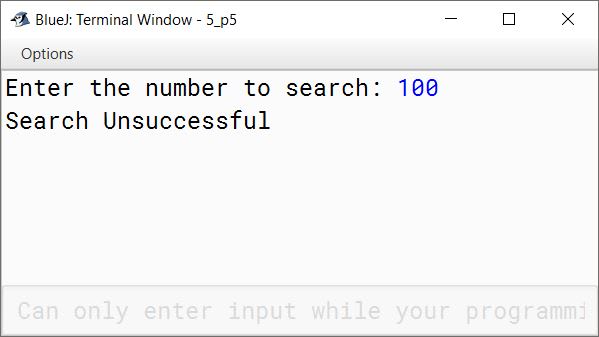
Answered By
18 Likes
Related Questions
A class teacher wants to arrange the names of her students in alphabetical order. Define a class NameSorter that stores the given names in a one-dimensional array. Sort the names in alphabetical order using Bubble Sort technique only and display the sorted names.
Aryan, Zoya, Ishaan, Neha, Rohan, Tanya, Manav, Simran, Kabir, Pooja
public class NameSorter { void bubbleSort(String names[]) { int len = names.length; _______(1)_________ { _______(2)_________ { _______(3)_________ { String t = names[j]; _______(4)_________ _______(5)_________ } } } } public static void main(String[] args) { String arr[] = {"Aryan", "Zoya", "Ishaan", "Neha", "Rohan", "Tanya", "Manav", "Simran", "Kabir", "Pooja" }; NameSorter obj = new NameSorter(); obj.bubbleSort(arr); for (int i = 0; i < arr.length; i++) { System.out.println(arr[i]); } } }
Define a class to accept values into 4x4 array and find and display the sum of each row.
Example:
A[][]={{1,2,3,4},{5,6,7,8},{1,3,5,7},{2,5,3,1}}
Output:
sum of row 1 = 10 (1+2+3+4) sum of row 2 = 26 (5+6+7+8) sum of row 3 = 16 (1+3+5+7) sum of row 4 = 11 (2+5+3+1)
Name the method of search depicted in the below picture:
Write a Java program to store n numbers in an one dimensional array. Pass this array to a function number(int a[]). Display only those numbers whose sum of digit is prime.