Computer Applications
A class teacher wants to arrange the names of her students in alphabetical order. Define a class NameSorter that stores the given names in a one-dimensional array. Sort the names in alphabetical order using Bubble Sort technique only and display the sorted names.
Aryan, Zoya, Ishaan, Neha, Rohan, Tanya, Manav, Simran, Kabir, Pooja
public class NameSorter
{
void bubbleSort(String names[]) {
int len = names.length;
_______(1)_________ {
_______(2)_________ {
_______(3)_________ {
String t = names[j];
_______(4)_________
_______(5)_________
}
}
}
}
public static void main(String[] args) {
String arr[] = {"Aryan", "Zoya",
"Ishaan", "Neha",
"Rohan", "Tanya",
"Manav", "Simran",
"Kabir", "Pooja"
};
NameSorter obj = new NameSorter();
obj.bubbleSort(arr);
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
Java Arrays
3 Likes
Answer
for (int i = 0; i < len - 1; i++)
for (int j = 0; j < len - 1 - i; j++)
if (names[j].compareToIgnoreCase(names[j + 1]) > 0)
names[j] = names[j + 1];
names[j + 1] = t;
Explanation
public class NameSorter
{
void bubbleSort(String names[]) {
int len = names.length;
for (int i = 0; i < len - 1; i++) {
for (int j = 0; j < len - 1 - i; j++) {
if (names[j].compareToIgnoreCase(names[j + 1]) > 0) {
String t = names[j];
names[j] = names[j + 1];
names[j + 1] = t;
}
}
}
}
public static void main(String[] args) {
String arr[] = {"Aryan", "Zoya",
"Ishaan", "Neha",
"Rohan", "Tanya",
"Manav", "Simran",
"Kabir", "Pooja"
};
NameSorter obj = new NameSorter();
obj.bubbleSort(arr);
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
Variable Description Table
Method bubbleSort
:
Variable Name | Data Type | Purpose |
---|---|---|
names | String[] | An array of Strings that holds the list of names to be sorted. |
len | int | The length of the names array, indicating the number of names to be sorted. |
i | int | An index variable used as a loop counter for the outer loop to control the number of passes. |
j | int | An index variable used as a loop counter for the inner loop to compare adjacent elements. |
t | String | A temporary variable used to hold a name value during the swapping process. |
Method main
:
Variable Name | Data Type | Purpose |
---|---|---|
arr | String[] | An array of Strings containing the names to be sorted. |
obj | NameSorter | An instance of the NameSorter class used to call the bubbleSort method. |
i | int | An index variable used as a loop counter to iterate over the sorted names for printing. |
Program Explanation
Let's analyze the Java program step by step to understand its functionality:
1. Class Definition:
- The class
NameSorter
is defined, which contains a methodbubbleSort
and themain
method to execute the program.
2. bubbleSort Method:
- The method
bubbleSort
accepts an array of stringsnames[]
as a parameter. This method will sort the strings in the array in alphabetical order.
3. Outer and Inner Loops:
- The
bubbleSort
uses two nested loops to iterate through the array:- The outer loop runs from the beginning of the array to the second-to-last element (
len - 1
). The variablei
serves as the counter for this loop. - The inner loop runs from the start of the array up to the last unsorted element (
len - 1 - i
). The variablej
is the counter for the inner loop. - The decreasing upper bound in the inner loop
len - 1 - i
is because with each complete pass through the array, the largest unsorted element gets correctly positioned at the end, reducing the number of elements needing comparison.
- The outer loop runs from the beginning of the array to the second-to-last element (
4. Comparison and Swapping:
- Inside the inner loop, a comparison is made between adjacent elements using
compareToIgnoreCase
:- The
compareToIgnoreCase
method is used to compare two strings lexicographically, returning a result based on whether the first string is considered greater, equal, or smaller than the second string, without regard to case. - If
names[j]
is lexicographically greater thannames[j + 1]
(i.e.,compareToIgnoreCase
returns a positive number), the elements are swapped. This ensures larger elements "bubble up" to their correct position.
- The
5. Swapping Logic:
- A temporary string
t
is used to facilitate the swapping ofnames[j]
andnames[j+1]
. The algorithm storesnames[j]
int
, assignsnames[j+1]
tonames[j]
, and finally assignst
tonames[j+1]
.
6. Main Method:
- The
main
method initializes an arrayarr
with several names in mixed order. - An instance
obj
of theNameSorter
class is created. - The
bubbleSort
method is called on theobj
instance, passing thearr
to be sorted. - After sorting, the program iterates through the sorted array and prints each name to the console, displaying the names in alphabetical order.
Output
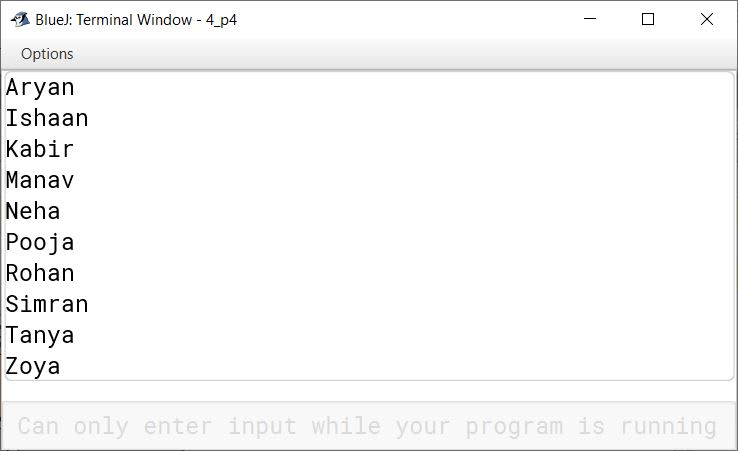
Answered By
3 Likes
Related Questions
Write a Java program to store n numbers in an one dimensional array. Pass this array to a function number(int a[]). Display only those numbers whose sum of digit is prime.
Consider the following two-dimensional array and answer the questions given below:
int x[ ][ ] = {{4,3,2}, {7,8,2}, {8, 3,10}, {1, 2, 9}};
(a) What is the order of the array?
(b) What is the value of x[0][0]+x[2][2]?
Define a class to accept values into an integer array of order 4 x 4 and check whether it is a DIAGONAL array or not. An array is DIAGONAL if the sum of the left diagonal elements equals the sum of the right diagonal elements. Print the appropriate message.
Example:
3 4 2 5
2 5 2 3
5 3 2 7
1 3 7 1Sum of the left diagonal element = 3 + 5 + 2 + 1 = 11
Sum of the right diagonal element = 5 + 2 + 3 + 1 = 11
Define a class to search for a value input by the user from the list of values given below. If it is found display the message "Search successful", otherwise display the message "Search element not found" using Binary search technique.
5.6, 11.5, 20.8, 35.4, 43.1, 52.4, 66.6, 78.9, 80.0, 95.5.