Computer Applications
Write a program to read the number n via the Scanner class and print the Tribonacci series:
0, 0, 1, 1, 2, 4, 7, 13, 24, 44, 81 …and so on.
Hint: The Tribonacci series is a generalisation of the Fibonacci sequence where each term is the sum of the three preceding terms.
Java
Java Iterative Stmts
14 Likes
Answer
import java.util.Scanner;
public class KboatTribonacci
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter no. of terms : ");
int n = in.nextInt();
if(n < 3)
System.out.print("Enter a number greater than 2");
else {
int a = 0, b = 0, c = 1;
System.out.print(a + " " + b + " " + c);
for (int i = 4; i <= n; i++) {
int term = a + b + c;
System.out.print(" " + term);
a = b;
b = c;
c = term;
}
}
}
}
Output
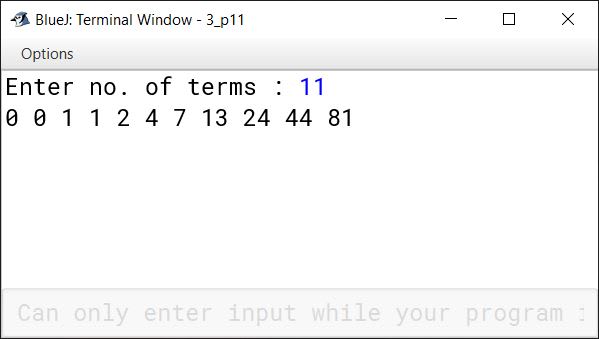
Answered By
3 Likes
Related Questions
Write a program to calculate the value of Pi with the help of the following series:
Pi = (4/1) - (4/3) + (4/5) - (4/7) + (4/9) - (4/11) + (4/13) - (4/15) …
Hint: Use while loop with 100000 iterations.
Write a menu driven program to display the pattern as per user’s choice.
Pattern 1
ABCDE
ABCD
ABC
AB
APattern 2
B
LL
UUU
EEEEFor an incorrect option, an appropriate error message should be displayed.
Write a program to accept n number of input integers and find out:
i. Number of positive numbers
ii. Number of negative numbers
iii. Sum of positive numbers
Write a program using do-while loop to compute the sum of the first 50 positive odd integers.