Computer Applications
Write a program to accept n number of input integers and find out:
i. Number of positive numbers
ii. Number of negative numbers
iii. Sum of positive numbers
Java
Java Iterative Stmts
11 Likes
Answer
import java.util.Scanner;
public class KboatIntegers
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int pSum = 0, pCount = 0, nCount = 0;
System.out.print("Enter the number of integers: ");
int num = in.nextInt();
System.out.println("Enter " + num + " integers: ");
for (int i = 1; i <= num; i++) {
int n = in.nextInt();
if (n >= 0) {
pSum += n;
pCount++;
}
else
nCount++;
}
System.out.println("Positive numbers = " + pCount);
System.out.println("Negative numbers = " + nCount);
System.out.println("Sum of positive numbers = " + pSum);
}
}
Output
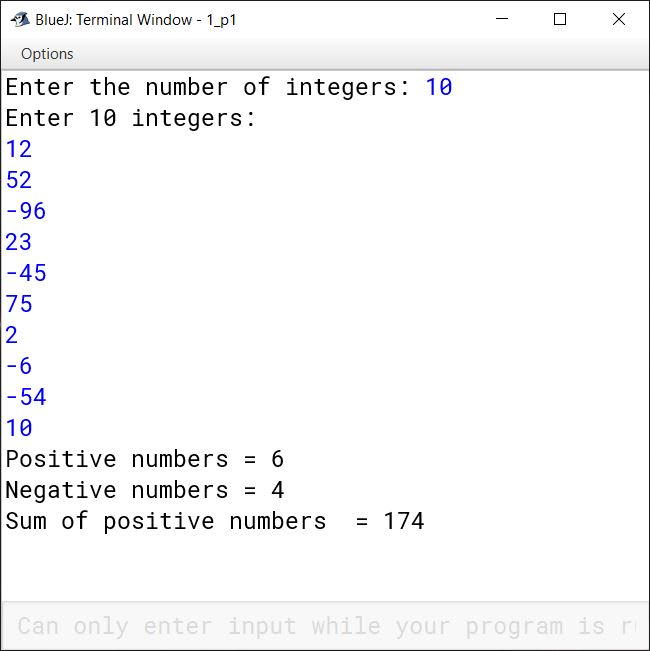
Answered By
4 Likes
Related Questions
Write a program to read the number n via the Scanner class and print the Tribonacci series:
0, 0, 1, 1, 2, 4, 7, 13, 24, 44, 81 …and so on.
Hint: The Tribonacci series is a generalisation of the Fibonacci sequence where each term is the sum of the three preceding terms.
How many times are the following loop bodies repeated? What is the final output in each case?
int z = 1; while (z < 10) if((z++) % 2 == 0) System.out.println(z);
Write a program using do-while loop to compute the sum of the first 50 positive odd integers.
How many times are the following loop bodies repeated? What is the final output in each case?
int y = 1; while (y < 10) if (y % 2 == 0) System.out.println(y++);