Computer Applications
Write a program to input two unequal numbers. Display the numbers after swapping their values in the variables without using a third variable.
Sample Input: a = 76, b = 65
Sample Output: a = 65, b = 76
Java
Input in Java
46 Likes
Answer
import java.util.Scanner;
public class KboatNumberSwap
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Please provide two unequal numbers");
System.out.print("Enter the first number: ");
int firstNum = in.nextInt();
System.out.print("Enter the second number: ");
int secondNum = in.nextInt();
if (firstNum == secondNum) {
System.out.println("Invalid Input. Numbers are equal.");
return;
}
firstNum = firstNum + secondNum;
secondNum = firstNum - secondNum;
firstNum = firstNum - secondNum;
System.out.println("First Number: " + firstNum);
System.out.println("Second Number: " + secondNum);
}
}
Variable Description Table
Program Explanation
Output
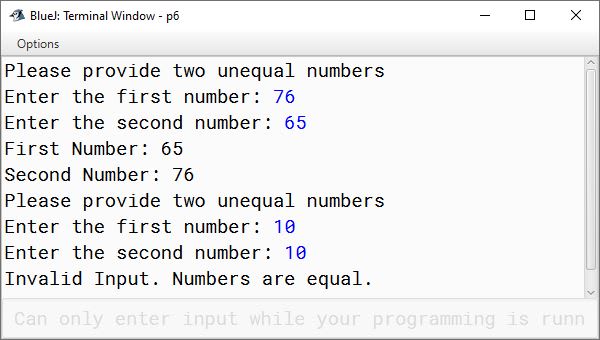
Answered By
22 Likes
Related Questions
The co-ordinates of two points A and B on a straight line are given as (x1,y1) and (x2,y2). Write a program to calculate the slope (m) of the line by using formula:
Slope = (y2 - y1) / (x2 - x1)
Take the co-ordinates (x1,y1) and (x2,y2) as input.
A certain amount of money is invested for 3 years at the rate of 6%, 8% and 10% per annum compounded annually. Write a program to calculate:
- the amount after 3 years.
- the compound interest after 3 years.
Accept certain amount of money (Principal) as an input.
Hint: A = P * (1 + (R1 / 100))T * (1 + (R2 / 100))T * (1 + (R3 / 100))T and CI = A - P
The driver took a drive to a town 240 km at a speed of 60 km/h. Later in the evening, he drove back at 20 km/h less than the usual speed. Write a program to calculate:
- the total time taken by the driver
- the average speed during the whole journey
[Hint: average speed = total distance / total time]
Write a program to input time in seconds. Display the time after converting them into hours, minutes and seconds.
Sample Input: Time in seconds: 5420
Sample Output: 1 Hour 30 Minutes 20 Seconds