Computer Applications
The co-ordinates of two points A and B on a straight line are given as (x1,y1) and (x2,y2). Write a program to calculate the slope (m) of the line by using formula:
Slope = (y2 - y1) / (x2 - x1)
Take the co-ordinates (x1,y1) and (x2,y2) as input.
Java
Input in Java
47 Likes
Answer
import java.util.Scanner;
public class KboatLineSlope
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter x coordinate of point A: ");
int x1 = in.nextInt();
System.out.print("Enter y coordinate of point A: ");
int y1 = in.nextInt();
System.out.print("Enter x coordinate of point B: ");
int x2 = in.nextInt();
System.out.print("Enter y coordinate of point B: ");
int y2 = in.nextInt();
float lineSlope = (y2 - y1) / (float)(x2 - x1);
System.out.println("Slope of line: " + lineSlope);
}
}
Variable Description Table
Program Explanation
Output
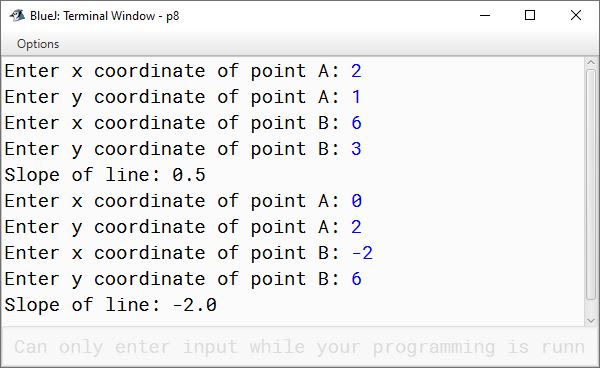
Answered By
23 Likes
Related Questions
Write a program to input two unequal numbers. Display the numbers after swapping their values in the variables without using a third variable.
Sample Input: a = 76, b = 65
Sample Output: a = 65, b = 76
The driver took a drive to a town 240 km at a speed of 60 km/h. Later in the evening, he drove back at 20 km/h less than the usual speed. Write a program to calculate:
- the total time taken by the driver
- the average speed during the whole journey
[Hint: average speed = total distance / total time]
A dealer allows his customers a discount of 25% and still gains 25%. Write a program to input the cost of an article and display its selling price and marked price.
Hint: SP = ((100 + p%) / 100) * CP and MP = (100 / (100 - d%)) * SP
A certain amount of money is invested for 3 years at the rate of 6%, 8% and 10% per annum compounded annually. Write a program to calculate:
- the amount after 3 years.
- the compound interest after 3 years.
Accept certain amount of money (Principal) as an input.
Hint: A = P * (1 + (R1 / 100))T * (1 + (R2 / 100))T * (1 + (R3 / 100))T and CI = A - P