Computer Applications
Write a program to input two unequal numbers. Display the numbers after swapping their values in the variables without using a third variable.
Sample Input: a=23, b= 56
Sample Output: a=56, b=23
Java
Input in Java
30 Likes
Answer
import java.io.*;
public class KboatNumberSwap
{
public static void main(String args[]) throws IOException {
InputStreamReader read = new InputStreamReader(System.in);
BufferedReader in = new BufferedReader(read);
System.out.print("Enter first number: ");
int a = Integer.parseInt(in.readLine());
System.out.print("Enter second number: ");
int b = Integer.parseInt(in.readLine());
a = a + b;
b = a - b;
a = a - b;
System.out.println("Numbers after Swap:");
System.out.println("a = " + a + "\t" + "b = " + b);
}
}
Output
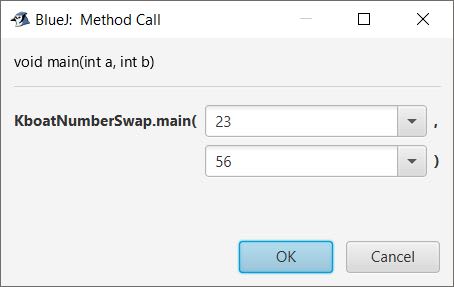
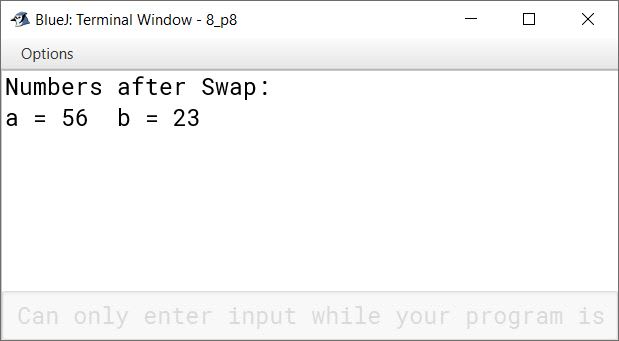
Answered By
15 Likes
Related Questions
Write a program to accept Principal, Rate and Time. Calculate and display the interest accumulated for the first year, second year and the third year compound annually.
Sample Input:
Principal = ₹5,000, Rate = 10% per annum, Time = 3 yearsSample Output:
Interest for the first year: ₹500
Interest for the second year: ₹550
Interest for the third year: ₹605A shopkeeper announces two successive discounts 20% and 10% on purchasing of goods on the marked price. Write a program to input marked price and calculate the selling price of an article.
Write a program to input the temperature in Celsius and convert it into Fahrenheit. If the temperature is more than 98.6 °F then display "Fever" otherwise "Normal".
Write a program to accept marks of a student obtained in 5 different subjects (English, Phy., Chem., Biology, Maths.) and find the average. If the average is 80% or more then he/she is eligible to get "Computer Science" otherwise "Biology".