Computer Applications
Write a program to accept Principal, Rate and Time. Calculate and display the interest accumulated for the first year, second year and the third year compound annually.
Sample Input:
Principal = ₹5,000, Rate = 10% per annum, Time = 3 years
Sample Output:
Interest for the first year: ₹500
Interest for the second year: ₹550
Interest for the third year: ₹605
Java
Input in Java
79 Likes
Answer
import java.io.*;
public class KboatInterest
{
public static void main(String args[]) throws IOException {
InputStreamReader read = new InputStreamReader(System.in);
BufferedReader in = new BufferedReader(read);
System.out.print("Enter Principal: ");
double p = Double.parseDouble(in.readLine());
System.out.print("Enter Rate: ");
double r = Double.parseDouble(in.readLine());
System.out.print("Enter Time: ");
int t = Integer.parseInt(in.readLine());
double i1 = p * r * 1 / 100.0;
double amt = p + i1;
System.out.println("Interest for the first year: " + i1);
double i2 = amt * r * 1 / 100.0;
amt = amt + i2;
System.out.println("Interest for the second year: " + i2);
double i3 = amt * r * 1 / 100.0;
amt = amt + i3;
System.out.println("Interest for the third year: " + i3);
}
}
Output
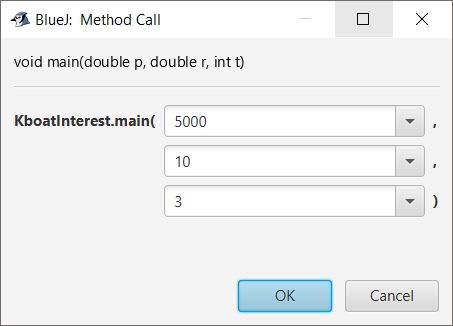
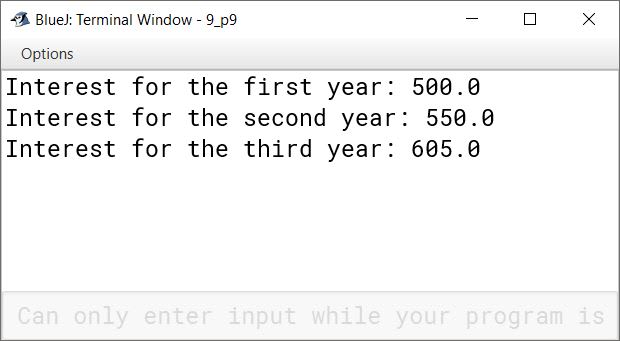
Answered By
42 Likes
Related Questions
A shopkeeper announces two successive discounts 20% and 10% on purchasing of goods on the marked price. Write a program to input marked price and calculate the selling price of an article.
Write a program to calculate the gross salary of an employee when
Basic Salary = Rs.8600
DA = 20% of Salary
HRA = 10% of Salary
CTA = 12% of the Salary.
Gross Salary = (Salary + DA + HRA + CTA)Write a program to input two unequal numbers. Display the numbers after swapping their values in the variables without using a third variable.
Sample Input: a=23, b= 56
Sample Output: a=56, b=23Write a program to calculate and display the value of the given expression:
(a2+b2)/(a-b),when a=20, b=15