Computer Applications
Write a program to input and store roll number and total marks of 20 students. Using Bubble Sort technique generate a merit list. Print the merit list in two columns containing roll number and total marks in the below format:
Roll No. Total Marks
… …
… …
… …
Java
Java Arrays
14 Likes
Answer
import java.util.Scanner;
public class MeritList
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int n = 20;
int rollNum[] = new int[n];
int marks[] = new int[n];
for (int i = 0; i < n; i++) {
System.out.print("Enter Roll Number: ");
rollNum[i] = in.nextInt();
System.out.print("Enter marks: ");
marks[i] = in.nextInt();
}
//Bubble Sort
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (marks[j] < marks[j + 1]) {
int t = marks[j];
marks[j] = marks[j+1];
marks[j+1] = t;
t = rollNum[j];
rollNum[j] = rollNum[j+1];
rollNum[j+1] = t;
}
}
}
System.out.println("Roll No.\t\tTotal Marks");
for (int i = 0; i < n; i++) {
System.out.println(rollNum[i] + "\t\t" + marks[i]);
}
}
}
Output
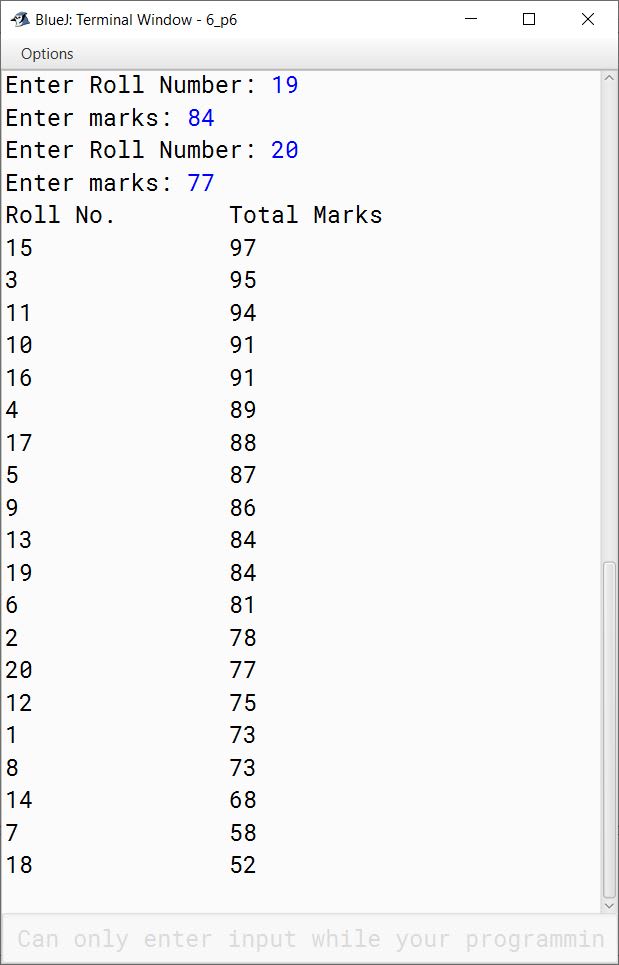
Answered By
4 Likes
Related Questions
Define a class pin code and store the given pin codes in a single dimensional array. Sort these pin codes in ascending order using the Selection Sort technique only. Display the sorted array.
110061, 110001, 110029, 110023, 110055, 110006, 110019, 110033
Name the method of search depicted in the below picture:
The statement used to find the total number of Strings present in the string array String s[] is:
- s.length
- s.length()
- length(s)
- len(s)
A single dimensional array contains 37 elements. Which of the following represents the index of its second-to-last element.