Computer Applications
Write a program to input and store integer elements in a double dimensional array of size 4 x 4 and find the sum of all the elements.
7 3 4 5
5 4 6 1
6 9 4 2
3 2 7 5
Sum of all the elements: 73
Java
Java Arrays
15 Likes
Answer
import java.util.Scanner;
public class KboatDDASum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[][] = new int[4][4];
long sum = 0;
System.out.println("Enter the elements of 4 x 4 DDA: ");
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
arr[i][j] = in.nextInt();
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
sum += arr[i][j];
}
}
System.out.println("Sum of all the elements = " + sum);
}
}
Output
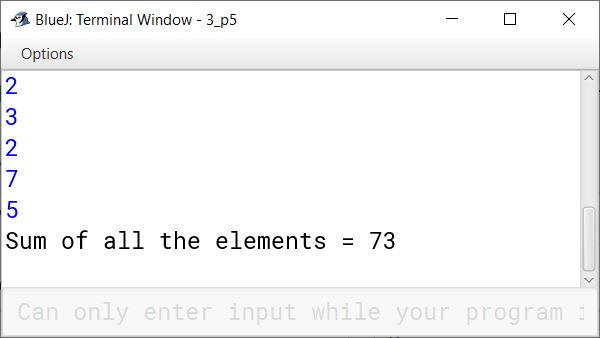
Answered By
8 Likes
Related Questions
Define a class to declare an array of size 20 of double datatype, accept the elements into the array and perform the following:
Calculate and print the sum of all the elements.
Calculate and print the highest value of the array.
Write a program to print following patterns.
(i)
1 2 3 4 5 1 2 3 4 1 2 3 1 2 1
(ii)
A B A C B A D C B A E D C B A
Write a program to input a number and check whether the number is an automorphic number or not.
An automorphic number is a number whose square "ends" in the same digits as the number itself.
e.g. 52 = 25, 62 = 36, 762 = 5776
Design a class RailwayTicket with following description:
Class name : RailwayTicket
Data Members Purpose String name To store the name of the customer String coach To store the type of coach customer wants to travel long mob no To store customer's mobile number int amt To store basic amount of ticket int totalamt To store the amount to be paid after updating the original amount Member Methods Purpose void accept() To take input for name, coach, mobile number and amount void update() To update the amount as per the coach selected (extra amount to be added in the amount as per the table below) void display() To display all details of a customer such as name, coach, total amount and mobile number Type of Coaches Amount First_AC ₹700 Second_AC ₹500 Third_AC ₹250 Sleeper None Write a main method to create an object of the class and call the above member methods.