Computer Applications
Write a program to input a set of 20 letters. Convert each letter into upper case. Find and display the number of vowels and number of consonants present in the set of given letters.
Java
Java Library Classes
105 Likes
Answer
import java.util.Scanner;
public class Kboat20LetterSet
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter any 20 letters");
int vc = 0, cc = 0;
for (int i = 0; i < 20; i++) {
char ch = in.next().charAt(0);
ch = Character.toUpperCase(ch);
if (ch == 'A' ||
ch == 'E' ||
ch == 'I' ||
ch == 'O' ||
ch == 'U')
vc++;
else if (ch >= 'A' && ch <= 'Z')
cc++;
}
System.out.println("Number of Vowels = " + vc);
System.out.println("Number of Consonants = " + cc);
}
}
Variable Description Table
Program Explanation
Output
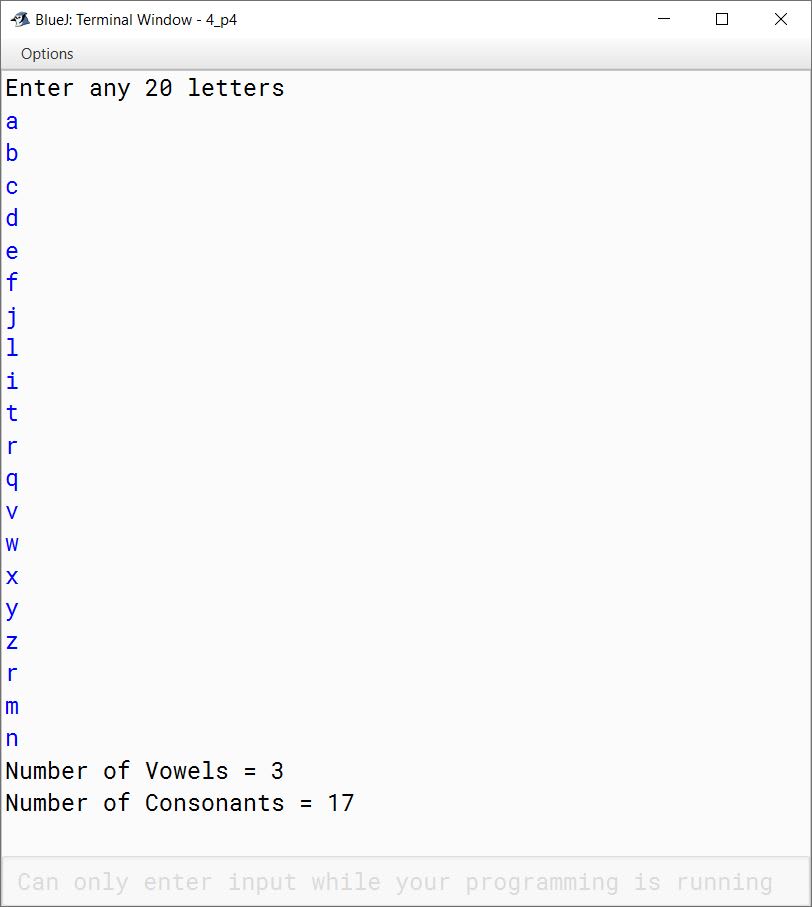
Answered By
48 Likes
Related Questions
Write a program in Java to input a character. Display next 5 characters.
Write a program in Java to accept an integer number N such that 0<N<27. Display the corresponding letter of the alphabet (i.e. the letter at position N).
[Hint: If N =1 then display A]Write a program in Java to generate all the alternate letters in the range of letters from A to Z.
Write a program to input two characters from the keyboard. Find the difference (d) between their ASCII codes. Display the following messages:
If d = 0 : both the characters are same.
If d \< 0 : first character is smaller.
If d > 0 : second character is smaller.
Sample Input :
D
P
Sample Output :
d = (68 - 80) = -12
First character is smaller